俄罗斯方块游戏:使用C语言实现一个简单的俄罗斯方块游戏。
时间: 2023-12-26 12:05:14 浏览: 137
好的,下面是一个简单的俄罗斯方块游戏的C语言实现。该游戏使用了简单的字符画来绘制游戏界面,并通过键盘输入来控制方块的移动和旋转。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#define WIDTH 10
#define HEIGHT 20
char screen[HEIGHT][WIDTH + 1];
int shape[7][4][4] = {
// I
{
{0, 0, 0, 0},
{1, 1, 1, 1},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// J
{
{2, 0, 0, 0},
{2, 2, 2, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// L
{
{0, 0, 3, 0},
{3, 3, 3, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// O
{
{4, 4, 0, 0},
{4, 4, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// S
{
{0, 5, 5, 0},
{5, 5, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// T
{
{0, 6, 0, 0},
{6, 6, 6, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// Z
{
{7, 7, 0, 0},
{0, 7, 7, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
}
};
int cur_x, cur_y, cur_shape, cur_rotation;
void init_game()
{
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
screen[i][j] = ' ';
}
screen[i][WIDTH] = '\0';
}
}
void draw_game()
{
int i;
system("cls");
for (i = 0; i < HEIGHT; i++) {
printf("%s\n", screen[i]);
}
}
void draw_shape(int x, int y, int shape, int rotation, char c)
{
int i, j;
for (i = 0; i < 4; i++) {
for (j = 0; j < 4; j++) {
if (shape[i][j]) {
screen[y + i][x + j] = c;
}
}
}
}
int check_collision(int x, int y, int shape, int rotation)
{
int i, j;
for (i = 0; i < 4; i++) {
for (j = 0; j < 4; j++) {
if (shape[i][j]) {
if (y + i >= HEIGHT || x + j < 0 || x + j >= WIDTH || screen[y + i][x + j] != ' ') {
return 1;
}
}
}
}
return 0;
}
void clear_full_rows()
{
int i, j, k;
for (i = HEIGHT - 1; i >= 0; i--) {
int full = 1;
for (j = 0; j < WIDTH; j++) {
if (screen[i][j] == ' ') {
full = 0;
break;
}
}
if (full) {
for (k = i; k > 0; k--) {
for (j = 0; j < WIDTH; j++) {
screen[k][j] = screen[k - 1][j];
}
}
for (j = 0; j < WIDTH; j++) {
screen[0][j] = ' ';
}
i++;
}
}
}
void new_shape()
{
cur_x = WIDTH / 2 - 2;
cur_y = 0;
cur_shape = rand() % 7;
cur_rotation = 0;
if (check_collision(cur_x, cur_y, shape[cur_shape], cur_rotation)) {
printf("Game over!\n");
exit(0);
}
}
void move_shape(int dx, int dy, int dr)
{
if (!check_collision(cur_x + dx, cur_y + dy, shape[cur_shape], (cur_rotation + dr) % 4)) {
cur_x += dx;
cur_y += dy;
cur_rotation = (cur_rotation + dr) % 4;
}
}
void game_loop()
{
int i, j, k;
int timer = 0;
while (1) {
if (_kbhit()) {
int ch = _getch();
switch (ch) {
case 'a':
move_shape(-1, 0, 0);
break;
case 'd':
move_shape(1, 0, 0);
break;
case 's':
move_shape(0, 1, 0);
break;
case 'w':
move_shape(0, 0, 1);
break;
case 'q':
move_shape(-1, 0, -1);
break;
case 'e':
move_shape(1, 0, 1);
break;
case 'p':
exit(0);
break;
}
}
timer++;
if (timer == 30) {
if (!check_collision(cur_x, cur_y + 1, shape[cur_shape], cur_rotation)) {
cur_y++;
} else {
draw_shape(cur_x, cur_y, shape[cur_shape], cur_rotation, 'X');
clear_full_rows();
new_shape();
}
timer = 0;
}
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
screen[i][j] = ' ';
}
screen[i][WIDTH] = '\0';
}
draw_shape(cur_x, cur_y, shape[cur_shape], cur_rotation, '*');
draw_game();
}
}
int main()
{
srand(time(NULL));
init_game();
new_shape();
game_loop();
return 0;
}
```
该游戏使用了一个 `screen` 数组来存储游戏界面,每个方格用一个字符表示。`shape` 数组存储了所有的方块形状,每个方块由四个小方块组成。
游戏循环会不断地检测用户输入,并根据输入移动或旋转当前方块。同时,每隔一定时间会让当前方块向下移动一格,直到碰到其他方块或游戏界面底部。
当一个方块无法继续向下移动时,会将其放置在游戏界面中,并清除满行。然后生成一个新的方块继续游戏。如果新生成的方块与当前方块重叠,则游戏结束。
阅读全文
相关推荐
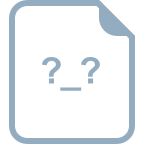
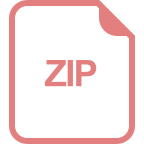















