立方体透视投影 实验代码 头歌
时间: 2023-07-09 13:04:56 浏览: 162
很抱歉,我不清楚你具体需要哪种编程语言的实验代码。不过,如果你需要立方体透视投影的实验代码,可以参考以下Python代码:
```python
import pygame
from pygame.locals import *
import math
# 定义常量
W, H = 800, 600 # 窗口大小
FOV = math.pi / 3 # 视角
HALF_W, HALF_H = W // 2, H // 2 # 窗口中心点
CUBE_SIZE = 100 # 立方体大小
# 定义立方体八个顶点
cube_points = [
(-1, -1, -1),
(-1, 1, -1),
(1, 1, -1),
(1, -1, -1),
(-1, -1, 1),
(-1, 1, 1),
(1, 1, 1),
(1, -1, 1)
]
# 定义立方体六个面,每个面是由四个点组成的tuple
cube_faces = [
(0, 1, 2, 3),
(4, 5, 6, 7),
(0, 1, 5, 4),
(2, 3, 7, 6),
(0, 3, 7, 4),
(1, 2, 6, 5)
]
# 定义旋转矩阵,绕x,y,z轴旋转的角度分别为rx,ry,rz
def rotation_matrix(rx, ry, rz):
cos_rx, sin_rx = math.cos(rx), math.sin(rx)
cos_ry, sin_ry = math.cos(ry), math.sin(ry)
cos_rz, sin_rz = math.cos(rz), math.sin(rz)
# 绕x轴旋转
rx_matrix = [
[1, 0, 0],
[0, cos_rx, -sin_rx],
[0, sin_rx, cos_rx]
]
# 绕y轴旋转
ry_matrix = [
[cos_ry, 0, sin_ry],
[0, 1, 0],
[-sin_ry, 0, cos_ry]
]
# 绕z轴旋转
rz_matrix = [
[cos_rz, -sin_rz, 0],
[sin_rz, cos_rz, 0],
[0, 0, 1]
]
return matmul(matmul(rz_matrix, ry_matrix), rx_matrix)
# 矩阵乘法
def matmul(a, b):
zip_b = list(zip(*b))
return [[sum(ele_a * ele_b for ele_a, ele_b in zip(row_a, col_b))
for col_b in zip_b] for row_a in a]
# 透视投影
def perspective_projection(point):
x, y, z = point
if z == 0:
z = 0.001 # 避免除以0
f = 1 / math.tan(FOV / 2)
k = W / 2
l = H / 2
return (int(k + x * f / z * k), int(l - y * f / z * l))
# 初始化pygame
pygame.init()
screen = pygame.display.set_mode((W, H))
pygame.display.set_caption("Cube Projection")
# 开始渲染循环
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
exit()
# 清空背景
screen.fill((255, 255, 255))
# 计算旋转矩阵
rx, ry, rz = 0, pygame.time.get_ticks() / 1000, 0
rot_matrix = rotation_matrix(rx, ry, rz)
# 渲染每个面
for face in cube_faces:
points = [cube_points[i] for i in face]
projected_points = []
for point in points:
# 应用旋转矩阵
rotated_point = matmul(rot_matrix, point)
# 透视投影
projected_point = perspective_projection(rotated_point)
projected_points.append(projected_point)
# 绘制面
pygame.draw.polygon(screen, (0, 0, 0), projected_points, 1)
# 刷新屏幕
pygame.display.flip()
```
这段代码使用了Pygame库来进行图形渲染,实现了立方体的透视投影,并可以对立方体进行旋转。你可以根据自己的需要修改代码,比如修改立方体大小、调整视角等。
阅读全文
相关推荐
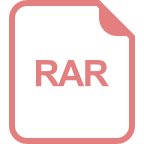
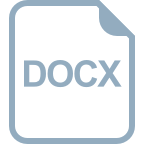
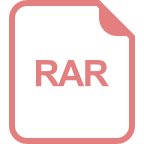















