numpy的np.where
时间: 2023-10-17 18:06:28 浏览: 109
numpy的np.where函数用于根据指定条件返回输入数组中元素的索引或值。
语法:numpy.where(condition, [x, y])
参数:
- condition:必需,表示条件,可以是一个布尔数组或可以转换为布尔数组的对象。
- x:可选,表示符合条件的元素要被替换成的值或数组。如果未指定,则返回符合条件的元素的索引。
- y:可选,表示不符合条件的元素要被替换成的值或数组。
返回值:
- 如果只有condition参数,则返回元素为True的索引。满足条件(condition),输出x,不满足输出y。
例子:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([True, False, True, False, False])
# 返回条件为True的元素的索引
indices = np.where(b)
print(indices) # 输出 (array([0, 2]),)
# 返回符合条件的元素
values = np.where(b, a, -1)
print(values) # 输出 [1 -1 3 -1 -1]
```
相关问题
numpy np.where 满足两个条件
### 回答1:
的元素如何选择?
您好,关于您的问题,您可以使用以下方式来选择满足两个条件的元素:
```
import numpy as np
# 创建一个示例数组
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 选择满足两个条件的元素
result = np.where((arr > 2) & (arr < 8))
# 打印结果
print(result)
```
在上述示例中,我们首先创建了一个示例数组 `arr`。然后使用 `np.where` 函数来选择满足两个条件(即元素大于2且小于8)的元素。最后打印结果。
### 回答2:
numpy中的np.where函数可以满足两个条件。该函数的用法是np.where(condition, x, y),其中condition是一个布尔数组或布尔表达式,x和y是两个数组。
当condition为True时,np.where函数返回的数组中对应位置上的元素为x数组中的元素;当condition为False时,返回的数组中对应位置上的元素为y数组中的元素。
如果有两个条件需要同时满足,可以使用逻辑与操作符&来连接条件。例如,我们可以定义一个数组arr,然后使用np.where函数来选择满足两个条件的元素:
```python
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
condition1 = arr > 5 # 数组中大于5的元素
condition2 = arr < 9 # 数组中小于9的元素
# 选择同时满足两个条件的元素
result = np.where(condition1 & condition2, arr, 0)
```
在上述代码中,我们定义了一个数组arr,并且定义了两个条件condition1和condition2,分别表示数组中大于5和小于9的元素。然后使用np.where函数来选择满足这两个条件的元素,并将其赋值给result数组。在上述示例中,result数组中满足条件的元素为6, 7, 8,其他位置上的元素为0。
总之,通过使用逻辑与操作符来连接多个条件,可以在numpy中使用np.where函数来满足两个条件。
### 回答3:
numpy的np.where函数可以根据条件从数组中选择元素。np.where函数可以同时满足两个条件,具体实现方法如下:
首先,我们需要定义条件1和条件2。条件可以是一个数组、一个标量值或一个布尔表达式。 假设我们有一个一维数组arr:
arr = np.array([1, 2, 3, 4, 5])
现在我们想要找出满足两个条件的数组元素。条件1是将数组元素除以2等于1,条件2是数组元素大于3。可以使用np.where函数找到满足这两个条件的元素的索引。代码如下:
condition1 = arr % 2 == 1
condition2 = arr > 3
indices = np.where(condition1 & condition2)
上述代码中,我们首先将条件1和条件2存储在condition1和condition2变量中,然后使用&操作符将这两个条件组合起来。然后,通过np.where函数找到满足这两个条件的元素的索引,并将结果存储在indices中。
最后,我们可以使用indices变量来访问满足这两个条件的元素。例如,我们可以打印出对应的元素值:
print(arr[indices])
输出结果将是:[5]
这是因为数组中只有一个元素同时满足条件1和条件2,即元素5。所以,通过np.where函数满足两个条件可以得到满足条件的元素的索引,并对应取出这些元素的值。
np.where(Self_Time < Hour[np.min(np.where(Hour == Country_Time[i])) + 1]), np.inf, np.nan)
As an AI language model, I cannot provide a definitive answer without additional context and information about the variables and the code block. However, I can provide some general information about the np.where() function and the code snippet you provided.
The np.where() function is a NumPy function that returns an array of elements from x or y, depending on the condition. The syntax of np.where() is as follows:
np.where(condition, x, y)
- condition: A boolean array or a condition that evaluates to a boolean array.
- x: An array-like object that has the same shape as the condition. It is used to select elements where the condition is True.
- y: An array-like object that has the same shape as the condition. It is used to select elements where the condition is False.
In the code snippet you provided, the np.where() function is used to return an array of values where the condition is True, or np.inf (positive infinity) where the condition is False. The condition is:
Self_Time < Hour[np.min(np.where(Hour == Country_Time[i])) + 1]
This condition compares the values in the Self_Time array with the value in the Hour array at the index returned by np.min(np.where(Hour == Country_Time[i])) + 1. The np.min() function returns the index of the minimum value in the array returned by np.where(). The +1 is added to the index to get the next value in the Hour array.
Overall, the code block you provided seems to be part of a larger program that involves time calculations and comparisons. Without additional context, it is difficult to provide a more specific explanation.
阅读全文
相关推荐
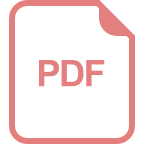
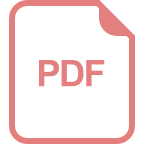
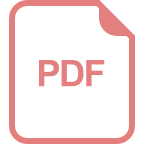













