如何用C语言编程来解析并求解一元二次方程的根?
时间: 2024-11-10 17:14:16 浏览: 22
在C语言中,你可以使用标准库函数`sqrt()`来计算平方根,并结合一些基本的数学操作来解决一元二次方程。假设我们有一个标准的一元二次方程形式 `ax^2 + bx + c = 0`,其中a、b和c是已知的系数。下面是一个简单的步骤:
1. 首先检查系数a是否为0,因为如果a=0,那么这不是一个二次方程而是线性方程。
2. 计算判别式`D = b^2 - 4ac`。判别式的值决定了根的性质:
- 如果`D > 0`,有两个实数根;
- 如果`D = 0`,有一个实数根(重根);
- 如果`D < 0`,有两个复数根。
3. 根据判别式的结果,计算两个根:
- sqrt(D)) / (2a)`。
- 复数根(如果需要):`x1 = (-b + i * sqrt(-D)) / (2a)` 和 `x2 = (-b - i * sqrt(-D)) / (2a)`,这里`i`是虚数单位。
以下是一个简单的C程序示例:
```c
#include <stdio.h>
#include <math.h>
void solveQuadratic(double a, double b, double c) {
double discriminant = b * b - 4 * a * c;
if (a == 0) {
printf("This is not a quadratic equation, it's linear.\n");
} else {
if (discriminant > 0) {
double root1 = (-b + sqrt(discriminant)) / (2 * a);
double root2 = (-b - sqrt(discriminant)) / (2 * a);
printf("Roots are: %.2lf and %.2lf\n", root1, root2);
} else if (discriminant == 0) {
double root = -b / (2 * a);
printf("There's one real root: %.2lf\n", root);
} else {
double realPart = -b / (2 * a);
double imaginaryPart = sqrt(-discriminant) / (2 * a);
printf("There are two complex roots: %.2lf + %.2lfi and %.2lf - %.2lfi\n",
realPart, imaginaryPart, realPart, imaginaryPart);
}
}
}
int main() {
double a, b, c;
printf("Enter coefficients a, b, and c of the quadratic equation: ");
scanf("%lf %lf %lf", &a, &b, &c);
solveQuadratic(a, b, c);
return 0;
}
```
阅读全文
相关推荐
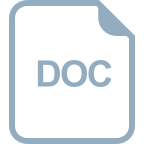
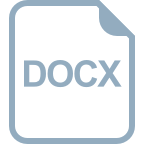
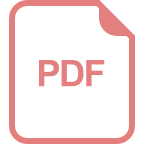















