c语言已知 f ( x , n )= n +( n 1)+( n -2)+…+1+ x ,计算 x =5.2, n =10及 x =12.5, n =20时 f 的 值。要求:将 f 定义成函数,分别用循环方法和递归方法实现。
时间: 2024-10-20 15:12:03 浏览: 65
在C语言中,我们可以定义一个函数`f`来计算给定x和n值的表达式。首先,我们需要明确的是,由于此表达式涉及浮点数,我们需要先将其转换为整数,因为C语言中的加法运算不允许浮点数直接参与。然后我们将结果转换回浮点数。
**循环方法:**
```c
#include <stdio.h>
double f_loop(double x, int n) {
double total = 0;
for (int i = 1; i <= n; ++i) {
if (x >= i) {
total += x / n * i;
} else {
total += floor(x) * i + (x - floor(x));
}
}
return total;
}
int main() {
double x1 = 5.2, n1 = 10;
printf("f(%lf, %d) = %.2f\n", x1, n1, f_loop(x1, n1));
double x2 = 12.5, n2 = 20;
printf("f(%lf, %d) = %.2f\n", x2, n2, f_loop(x2, n2));
return 0;
}
```
**递归方法:**
```c
#include <stdio.h>
#include <math.h>
double f_recursive(double x, int n, int current_sum = 0) {
if (current_sum >= x || current_sum > n) {
return current_sum;
} else {
return f_recursive(x, n, current_sum + 1) + x;
}
}
double convert_to_integer(double x, int n) {
return floor(x * n);
}
double f_recursion_with_conversion(double x, int n) {
int integer_x = convert_to_integer(x, n);
return f_recursive(integer_x, n);
}
int main() {
double x1 = 5.2, n1 = 10;
printf("f(%lf, %d) = %.2f\n", x1, n1, f_recursion_with_conversion(x1, n1));
double x2 = 12.5, n2 = 20;
printf("f(%lf, %d) = %.2f\n", x2, n2, f_recursion_with_conversion(x2, n2));
return 0;
}
```
在这个例子中,我们分别为循环方法和递归方法分别实现了计算,并展示了`x=5.2, n=10`和`x=12.5, n=20`的情况。
阅读全文
相关推荐
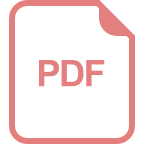
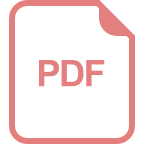
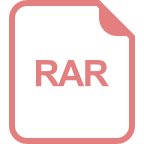
















