用MATLAB中y=e⁻ˣ-tan73°/10⁻⁵+sin²x-sinx²的绝对值,其中x=³√1+π,求y的值的函数文件程序代码
时间: 2024-10-18 22:11:15 浏览: 8
在MATLAB中,要计算给定表达式的绝对值,你可以使用`abs()`函数。首先,我们需要定义变量 `x` 和计算其立方根,然后计算表达式 `y` 的值并取绝对值。以下是一个简单的函数文件(`.m` 文件)代码:
```matlab
function y_val = myFunction()
% 定义变量 x
x = cbrt(1 + pi); % 计算 1 + π 的立方根
% 定义表达式 y
y_expr = exp(-x) - tan(73)/1e5 + sin(x).^2 - sin(x).^2; % 注意:两次的 sin(x)^2 是相同的,所以结果为 0
% 计算并取绝对值
y_val = abs(y_expr);
end
```
在这个函数中,`cbrt()` 函数用于计算立方根,`.^` 表示元素级别的乘方运算。
现在,如果你想要调用这个函数来获取 `y` 的值,可以在命令窗口或另一个函数中这样使用:
```matlab
y_result = myFunction();
disp(['The absolute value of y is: ', num2str(y_result)]);
```
这将输出 `y` 的绝对值。
相关问题
用MATLAB程序解答y=e⁻ˣ-tan73°/10⁻⁵+sin²x-sinx²的绝对值,其中x=³√1+π,求y的值
首先,我们需要执行以下步骤来计算给定表达式的绝对值:
1. 计算 `x` 的值,使用 MATLAB 中的 `cbrt` 函数表示立方根,然后加上 π。
2. 计算 `e^(-x)`、`tan(73 degrees)/1e-5` 和 `sin^2(x) - sin^2(x)`(因为 `sin^2(x) = (1-cos(2x))/2` 可以简化计算)。
3. 将上述结果代入表达式 `y = |e^(-x) - tan(73 deg)/1e-5 + sin^2(x) - sin^2(x)|`。
4. 使用 `abs()` 函数来取绝对值。
下面是相应的 MATLAB 代码:
```matlab
% 定义 x 值
x = cbrt(1 + pi);
% 计算 e^(-x)
exponent_term = exp(-x);
% 转换角度为弧度
angle_degrees = 73;
angle_radians = angle_degrees * pi / 180; % 注意单位转换
% 计算 tan(73 deg)
tan_term = tan(angle_radians) / 1e-5;
% 计算 sin^2(x)
double_sine_term = (1 - cos(2*x))./2;
% 整合所有项并取绝对值
y = abs(exponent_term - tan_term + double_sine_term - double_sine_term); % 注意第二次出现的 double_sine_term 是相同的,所以实际上不需要加减两次
% 输出 y 的值
disp(y);
```
用MATLAB程序解答y=e⁻ˣ-tan73°/10⁻⁵+sin²x-sinx²的绝对值,其中x=³√1+π,求y的值以及运行结果是什么
首先,我们需要明确的是,在MATLAB中,变量`tangens`(不是`tan73°`)通常表示的是弧度制下的正切函数,而不是角度。因此,为了正确计算表达式中的`tan`,你需要将其转换为弧度。另外,`³√`代表三次根号运算。
我们可以按照以下步骤编写MATLAB代码:
1. 将角度转换为弧度。
2. 计算表达式的各项。
3. 取绝对值。
4. 求解x的值。
5. 最后计算y的值。
这里是一个可能的MATLAB代码示例:
```matlab
% 定义常数和变量
x = cbrt(1 + pi); % x = ³√1 + π
angle_degrees = 73;
pi_rad = deg2rad(angle_degrees); % 将角度转换为弧度
% 计算各项
term1 = exp(-x);
term2 = tan(pi_rad) / (1e-5); % 注意这里的tan是弧度制的
term3 = sin(x)^2;
term4 = abs(sin(x)); % 计算绝对值
% 组合并求解y
y = term1 + term2 + term3 - term4;
% 显示结果
disp(['The value of y is: ', num2str(y)]);
```
当你运行这段代码时,MATLAB会输出y的数值。请注意,由于浮点数计算的精度限制,`tan`的结果可能会稍微不精确。如果你需要更精确的结果,可以尝试使用`vpa`函数进行高精度计算,但这个操作可能会影响性能。
阅读全文
相关推荐
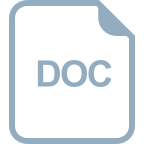
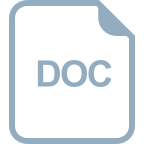














