用c语言写一个六子棋的代码
时间: 2023-08-13 19:04:22 浏览: 87
以下是一个简单的 C 语言六子棋游戏的代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define BOARD_SIZE 6 // 棋盘大小
char board[BOARD_SIZE][BOARD_SIZE]; // 棋盘
// 初始化棋盘
void init_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = '.';
}
}
}
// 显示棋盘
void print_board() {
printf("\n");
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
printf("%c ", board[i][j]);
}
printf("\n");
}
printf("\n");
}
// 判断是否胜利
bool is_win(char c) {
// 判断列
for (int i = 0; i < BOARD_SIZE; i++) {
int count = 0;
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[j][i] == c) {
count++;
if (count >= 4) {
return true;
}
} else {
count = 0;
}
}
}
// 判断行
for (int i = 0; i < BOARD_SIZE; i++) {
int count = 0;
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == c) {
count++;
if (count >= 4) {
return true;
}
} else {
count = 0;
}
}
}
// 判断正斜线
for (int i = 0; i <= BOARD_SIZE - 4; i++) {
for (int j = 0; j <= BOARD_SIZE - 4; j++) {
if (board[i][j] == c && board[i+1][j+1] == c &&
board[i+2][j+2] == c && board[i+3][j+3] == c) {
return true;
}
}
}
// 判断反斜线
for (int i = 0; i <= BOARD_SIZE - 4; i++) {
for (int j = BOARD_SIZE - 1; j >= 3; j--) {
if (board[i][j] == c && board[i+1][j-1] == c &&
board[i+2][j-2] == c && board[i+3][j-3] == c) {
return true;
}
}
}
return false;
}
// 下棋
bool play(int x, int y, char c) {
if (board[x][y] != '.') {
return false;
}
board[x][y] = c;
return true;
}
int main() {
init_board();
print_board();
char player1 = 'X';
char player2 = 'O';
int x, y;
char current_player = player1;
while (true) {
printf("Player %c's turn.\n", current_player);
printf("Enter row and column (e.g. 1 2): ");
scanf("%d %d", &x, &y);
if (play(x-1, y-1, current_player)) {
print_board();
if (is_win(current_player)) {
printf("Player %c wins!\n", current_player);
break;
}
if (current_player == player1) {
current_player = player2;
} else {
current_player = player1;
}
} else {
printf("Invalid move, try again.\n");
}
}
return 0;
}
```
该代码实现了一个简单的六子棋游戏,包括初始化棋盘,显示棋盘,下棋,判断胜利等功能。玩家可以通过输入行和列来下棋,程序会判断该位置是否可下,如果可下,则将该位置赋为当前玩家的棋子,并检查当前玩家是否胜利。如果胜利,则输出胜利信息并结束游戏。如果当前玩家不是胜利者,则交换玩家并继续游戏。
阅读全文
相关推荐
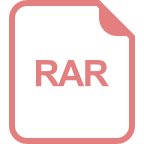
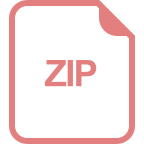
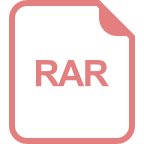















