easyx读取坐标点文件绘制图形
时间: 2024-01-11 20:03:42 浏览: 89
可以通过以下步骤使用EasyX读取坐标点文件并绘制图形:
1. 打开文件并读取坐标点数据,例如txt文件中每行格式为"x y",可以使用ifstream和getline函数来读取数据。
```c++
ifstream f("data.txt");
string line;
while (getline(f, line))
{
istringstream iss(line);
int x, y;
iss >> x >> y;
// 存储坐标数据
}
f.close();
```
2. 创建绘图窗口并设置绘图参数,例如背景色、坐标轴等。
```c++
initgraph(width, height);
setbkcolor(WHITE);
setlinecolor(BLACK);
setlinestyle(PS_SOLID, 1);
settextstyle(16, 0, _T("宋体"));
```
3. 绘制坐标轴及其他辅助线,例如x轴和y轴。
```c++
line(0, height / 2, width, height / 2); // x轴
line(width / 2, 0, width / 2, height); // y轴
```
4. 遍历坐标点数据并将其转换为屏幕坐标,然后使用line函数或者circle函数绘制图形。
```c++
for (int i = 0; i < points.size(); i++)
{
int x = points[i].x + width / 2;
int y = height / 2 - points[i].y;
circle(x, y, 2);
}
```
5. 关闭绘图窗口。
```c++
closegraph();
```
完整代码如下:
```c++
#include <graphics.h>
#include <fstream>
#include <sstream>
#include <vector>
using namespace std;
struct Point
{
int x;
int y;
};
int main()
{
int width = 600;
int height = 600;
vector<Point> points;
ifstream f("data.txt");
string line;
while (getline(f, line))
{
istringstream iss(line);
int x, y;
iss >> x >> y;
points.push_back({ x, y });
}
f.close();
initgraph(width, height);
setbkcolor(WHITE);
setlinecolor(BLACK);
setlinestyle(PS_SOLID, 1);
settextstyle(16, 0, _T("宋体"));
line(0, height / 2, width, height / 2);
line(width / 2, 0, width / 2, height);
for (int i = 0; i < points.size(); i++)
{
int x = points[i].x + width / 2;
int y = height / 2 - points[i].y;
circle(x, y, 2);
}
closegraph();
return 0;
}
```
阅读全文
相关推荐
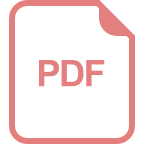
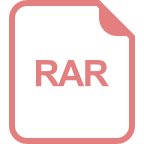
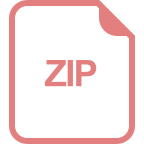














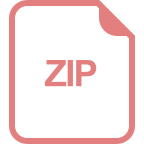
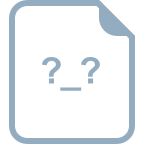