学生成绩查询 编写程序完成学生成绩记录的查询。 要求:按学号进行顺序查找;按学号排序后对学号进行折半查找; 随机输入以学号为关键字的学生信息并构建二叉排序树,对学号进行二叉排序树查找。
时间: 2024-03-01 16:51:11 浏览: 102
以下是一个实现上述要求的C++程序示例。该程序可以让用户选择不同的查询方式来查询学生成绩记录。
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
struct Student {
string id;
string name;
int score;
};
// 按学号进行顺序查找
bool searchById(vector<Student>& students, const string& id, int& score) {
for (const auto& student : students) {
if (student.id == id) {
score = student.score;
return true;
}
}
return false;
}
// 按学号排序后对学号进行折半查找
bool binarySearchById(vector<Student>& students, const string& id, int& score) {
sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.id < b.id;
});
int left = 0, right = students.size() - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (students[mid].id == id) {
score = students[mid].score;
return true;
} else if (students[mid].id < id) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return false;
}
// 构建二叉排序树
struct Node {
string id;
int score;
Node* left = nullptr;
Node* right = nullptr;
};
void insert(Node*& root, const Student& student) {
if (!root) {
root = new Node{student.id, student.score};
} else if (student.id < root->id) {
insert(root->left, student);
} else {
insert(root->right, student);
}
}
// 对学号进行二叉排序树查找
bool searchById(Node* root, const string& id, int& score) {
if (!root) {
return false;
}
if (root->id == id) {
score = root->score;
return true;
} else if (id < root->id) {
return searchById(root->left, id, score);
} else {
return searchById(root->right, id, score);
}
}
int main() {
vector<Student> students{
{"1001", "Tom", 80},
{"1002", "Jerry", 90},
{"1003", "Alice", 85},
{"1004", "Bob", 75}
};
int choice;
while (true) {
cout << "请选择查询方式 (1-顺序查找, 2-折半查找, 3-二叉排序树查找, 0-退出):" << endl;
cin >> choice;
if (choice == 0) {
break;
}
string id;
int score;
switch (choice) {
case 1:
cout << "请输入要查询的学生学号:" << endl;
cin >> id;
if (searchById(students, id, score)) {
cout << "学生 " << id << " 的成绩是:" << score << endl;
} else {
cout << "找不到学生 " << id << endl;
}
break;
case 2:
cout << "请输入要查询的学生学号:" << endl;
cin >> id;
if (binarySearchById(students, id, score)) {
cout << "学生 " << id << " 的成绩是:" << score << endl;
} else {
cout << "找不到学生 " << id << endl;
}
break;
case 3:
Node* root = nullptr;
for (const auto& student : students) {
insert(root, student);
}
cout << "请输入要查询的学生学号:" << endl;
cin >> id;
if (searchById(root, id, score)) {
cout << "学生 " << id << " 的成绩是:" << score << endl;
} else {
cout << "找不到学生 " << id << endl;
}
break;
default:
cout << "无效的选项" << endl;
break;
}
}
return 0;
}
```
在这个示例程序中,我们定义了一个 `Student` 结构体来表示学生的信息,包括学号、姓名和成绩。我们使用了一个 `vector` 容器来存储所有的学生信息,并在程序中添加了一些示例数据。
然后,我们定义了三种不同的查询方式:顺序查找、折半查找和二叉排序树查找。在顺序查找和折半查找中,我们使用了 `vector` 容器中的 `searchById` 和 `binarySearchById` 函数来查找指定学生的成绩。在二叉排序树查找中,我们使用了一个 `Node` 结构体来构建二叉排序树,并使用 `searchById` 函数来查找指定学生的成绩。
最后,我们在主函数中使用一个循环来让用户选择不同的查询方式,并根据用户的选择来执行相应的查询。
阅读全文
相关推荐
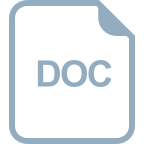
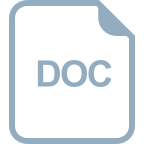
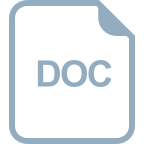












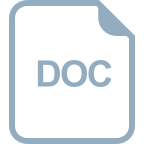
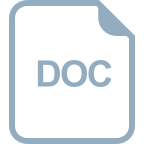