c语言贪吃蛇完整代码
时间: 2024-06-30 11:00:18 浏览: 182
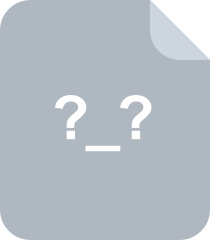
c语言贪吃蛇原代码.c
这份c语言贪吃蛇完整代码使用链表制作,包含两种游戏模式,代码简单,全文771行,部分功能阉割后代码可以更简洁,游戏流畅,暂时没发现bug,可以用作c语言课程设计。以下是代码的主要结构和功能:
1.头文件与全局变量
2.用来表示蛇与食物的结构体
3.初始化函数
4.绘制函数
5.移动函数
6.检测函数
7.游戏循环函数
8.主函数
完整代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#include <time.h>
#define WIDTH 40
#define HEIGHT 20
#define SPEED 150
typedef struct Node{
int x;
int y;
struct Node* next;
}node;
node* head;
node* food;
int score;
int mode;
void init();
void draw();
void move();
int check();
void gameLoop();
int main(){
init();
gameLoop();
return 0;
}
void init(){
srand((unsigned)time(NULL));
head = (node*)malloc(sizeof(node));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
node* body = (node*)malloc(sizeof(node));
body->x = head->x - 1;
body->y = head->y;
body->next = head;
head->next = body;
food = (node*)malloc(sizeof(node));
food->x = rand() % (WIDTH - 2) + 1;
food->y = rand() % (HEIGHT - 2) + 1;
food->next = NULL;
score = 0;
mode = 1;
}
void draw(){
system("cls");
for (int i = 0; i < WIDTH + 2; i++){
printf("#");
}
printf("\n");
for (int i = 0; i < HEIGHT; i++){
printf("#");
for (int j = 0; j < WIDTH; j++){
if (i == head->y && j == head->x){
printf("O");
}
else if (i == food->y && j == food->x){
printf("F");
}
else{
node* p = head->next;
int flag = 0;
while (p != NULL){
if (i == p->y && j == p->x){
printf("o");
flag = 1;
break;
}
p = p->next;
}
if (flag == 0){
printf(" ");
}
}
}
printf("#\n");
}
for (int i = 0; i < WIDTH + 2; i++){
printf("#");
}
printf("\n");
printf("Score: %d\n", score);
}
void move(){
int x = head->x;
int y = head->y;
int px, py;
node* p = head->next;
while (p != NULL){
px = p->x;
py = p->y;
p->x = x;
p->y = y;
x = px;
y = py;
p = p->next;
}
if (mode == 1){
switch (_getch()){
case 'w':
head->y--;
break;
case 's':
head->y++;
break;
case 'a':
head->x--;
break;
case 'd':
head->x++;
break;
}
}
else{
if (food->x > head->x){
head->x++;
}
else if (food->x < head->x){
head->x--;
}
else if (food->y > head->y){
head->y++;
}
else if (food->y < head->y){
head->y--;
}
}
}
int check(){
if (head->x == 0 || head->x == WIDTH - 1 || head->y == 0 || head->y == HEIGHT - 1){
return 1;
}
node* p = head->next;
while (p != NULL){
if (head->x == p->x && head->y == p->y){
return 1;
}
p = p->next;
}
if (head->x == food->x && head->y == food->y){
node* body = (node*)malloc(sizeof(node));
body->x = head->x;
body->y = head->y;
body->next = head->next;
head->next = body;
food->x = rand() % (WIDTH - 2) + 1;
food->y = rand() % (HEIGHT - 2) + 1;
score++;
}
return 0;
}
void gameLoop(){
while (1){
draw();
move();
if (check() == 1){
printf("Game Over!\n");
break;
}
Sleep(SPEED);
}
}
```
阅读全文
相关推荐
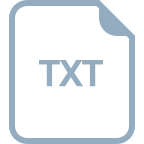















