igh ethercat程序汇川示例
时间: 2023-08-23 15:04:37 浏览: 137
以下是汇川EtherCAT控制器的示例程序,用于控制伺服驱动器:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "EcatFunction.h"
#include "SystemDefine.h"
#define EC_TIMEOUTMON 500
int main(int argc, char *argv[])
{
int cycle_init = 0, slv_num, slave;
unsigned int i, j;
char *ifname;
int expectedWKC;
int oloop, iloop, iodepth;
int run, chk, oob;
unsigned int ob;
unsigned int obcnt;
unsigned int obtimeout;
int ix;
// 初始化EtherCAT主站
if (argc > 1)
ifname = argv[1];
else
ifname = (char*)"eth0";
if (argc > 2)
cycle_init = atoi(argv[2]);
if (ec_init(ifname))
{
printf("EtherCAT initialized failed.\n");
return 0;
}
printf("EtherCAT initialized success.\n");
if (cycle_init)
ec_config_init(FALSE);
// 扫描所有从站
ec_config_map(&IOmap);
ec_configdc();
// 输出从站数量
slv_num = ec_slavecount;
printf("Slaves found : %d\n", slv_num);
// 输出所有从站的信息
for (i = 1; i <= ec_slavecount; i++)
{
printf("Slave %d\n", i);
printf("\tVendor: %s\n", ec_slave[i].eep_man);
printf("\tProduct: %s\n", ec_slave[i].eep_id);
printf("\tRevision: %s\n", ec_slave[i].eep_rev);
printf("\tPosition: %d\n", ec_slave[i].position);
printf("\tName: %s\n", ec_slave[i].name);
printf("\tOutputs: %d bytes (%d bits)\n", ec_slave[i].Obits / 8, ec_slave[i].Obits);
printf("\tInputs: %d bytes (%d bits)\n", ec_slave[i].Ibits / 8, ec_slave[i].Ibits);
printf("\tState: %d\n", ec_slave[i].state);
printf("\tDelay (ns): %d\n", ec_slave[i].pdelay);
}
// 使能所有从站
ec_statecheck(0, EC_STATE_SAFE_OP, EC_TIMEOUTSTATE);
if (ec_slave[0].state != EC_STATE_SAFE_OP)
{
printf("Not all slaves reached safe operational state.\n");
ec_readstate();
for (i = 1; i <= ec_slavecount; i++)
{
if (ec_slave[i].state != EC_STATE_SAFE_OP)
{
printf("Slave %d State=0x%2.2x StatusCode=0x%4.4x : %s\n", i, ec_slave[i].state, ec_slave[i].ALstatuscode, ec_ALstatuscode2string(ec_slave[i].ALstatuscode));
}
}
}
else
{
printf("All slaves reached safe operational state.\n");
}
// 运行主循环
run = TRUE;
for (iloop = 0; iloop < 1000000 && run; iloop++)
{
ec_send_processdata();
wkc = ec_receive_processdata(EC_TIMEOUTRET);
ec_statecheck(0, EC_STATE_SAFE_OP, EC_TIMEOUTSTATE);
// 读取从站状态
chk = 0;
for (slave = 1; slave <= ec_slavecount; slave++)
{
if (ec_slave[slave].state != EC_STATE_SAFE_OP)
{
printf("Slave %d State=0x%2.2x StatusCode=0x%4.4x : %s\n", slave, ec_slave[slave].state, ec_slave[slave].ALstatuscode, ec_ALstatuscode2string(ec_slave[slave].ALstatuscode));
chk = 1;
}
}
if (chk)
{
printf("EtherCAT is down!\n");
run = FALSE;
continue;
}
// 写入数据到从站
oob = 0;
obtimeout = 0;
obcnt = 0;
for (slave = 1; slave <= ec_slavecount; slave++)
{
if (ec_slave[slave].outputs > 0)
{
if (oob == 0)
{
printf("Requesting OP\n");
ec_send_processdata();
oob = 1;
}
ob = ec_slave[slave].outputs;
obcnt += ob;
ec_send_slave(slave, &ob, sizeof(ob));
}
}
if (oob)
{
expectedWKC = (ec_group[0].outputsWKC * 2) + ec_group[0].inputsWKC;
if (wkc < expectedWKC)
{
printf("Calculated workcounter %d, expected %d\n", wkc, expectedWKC);
}
ec_receive_processdata(EC_TIMEOUTRET);
oob = 0;
}
// 读取数据从从站
iodepth = 0;
for (slave = 1; slave <= ec_slavecount; slave++)
{
if (ec_slave[slave].inputs > 0)
{
iodepth += ec_slave[slave].inputs;
ec_receive_slave(slave, &ib, sizeof(ib));
printf("Slave %d Input Byte: %d\n", slave, ib);
}
}
}
// 关闭EtherCAT主站
ec_close();
printf("End of Program\n");
return (0);
}
```
这个示例程序使用了 EtherCAT主站API(EC-Master API) 库,包括了 EtherCAT 主站的配置、扫描从站、使能从站、发送和接收数据等操作。您需要根据实际情况修改配置和数据处理部分的代码。
相关推荐
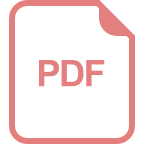
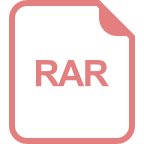














