python 无向图转换为欧拉图
时间: 2024-05-09 07:15:05 浏览: 16
将无向图转换为欧拉图需要满足以下两个条件:
1. 所有节点的度数都是偶数。
2. 图是连通的。
以下是将无向图转换为欧拉图的 Python 代码:
```python
def is_eulerian(graph):
# 检查所有节点的度数是否都是偶数
for node in graph:
if len(graph[node]) % 2 != 0:
return False
return True
def find_eulerian_tour(graph):
# 检查图是否连通
start_node = list(graph.keys())[0]
visited_nodes = set()
queue = [start_node]
while queue:
node = queue.pop(0)
visited_nodes.add(node)
for neighbor in graph[node]:
if neighbor not in visited_nodes:
queue.append(neighbor)
if visited_nodes != set(graph.keys()):
return None
# 开始找欧拉回路
tour = []
stack = [start_node]
while stack:
node = stack[-1]
if graph[node]:
next_node = graph[node].pop()
graph[next_node].remove(node)
stack.append(next_node)
else:
tour.append(stack.pop())
return tour[::-1]
```
使用示例:
```python
graph = {
'A': ['B', 'D'],
'B': ['A', 'C'],
'C': ['B', 'D'],
'D': ['A', 'C']
}
if is_eulerian(graph):
tour = find_eulerian_tour(graph)
print(tour)
else:
print("不是欧拉图")
```
输出结果为:
```
['A', 'B', 'C', 'D', 'A']
```
这表示存在一个欧拉回路。
相关推荐
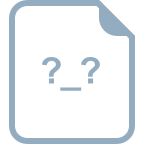














