unity webgl与java连接通信的方式和代码,unity通过http发送请求给java,获得java接口数据的方式和diam
时间: 2024-02-23 09:00:24 浏览: 175
Unity WebGL可以通过JavaScript与网页进行通信,而Java与Unity WebGL的通信可以使用WebSocket、HTTP等其他技术。下面是一种基于HTTP的通信方式的示例代码,演示了如何在Unity WebGL中使用HTTP与Java接口进行通信:
在Unity中,创建一个新的C#脚本,并使用以下代码:
```
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using UnityEngine.Networking;
public class JavaInterface : MonoBehaviour
{
private const string URL = "http://localhost:8080/api/"; // Java接口URL
// 发送HTTP GET请求
public IEnumerator GetRequest(string path, Dictionary<string, string> headers, System.Action<string> callback)
{
UnityWebRequest request = UnityWebRequest.Get(URL + path);
foreach (KeyValuePair<string, string> header in headers)
{
request.SetRequestHeader(header.Key, header.Value);
}
yield return request.SendWebRequest();
if (request.isNetworkError || request.isHttpError)
{
Debug.LogError(request.error);
}
else
{
callback(request.downloadHandler.text);
}
}
// 发送HTTP POST请求
public IEnumerator PostRequest(string path, Dictionary<string, string> headers, byte[] body, System.Action<string> callback)
{
UnityWebRequest request = UnityWebRequest.Post(URL + path, "");
foreach (KeyValuePair<string, string> header in headers)
{
request.SetRequestHeader(header.Key, header.Value);
}
request.uploadHandler = new UploadHandlerRaw(body);
request.downloadHandler = new DownloadHandlerBuffer();
yield return request.SendWebRequest();
if (request.isNetworkError || request.isHttpError)
{
Debug.LogError(request.error);
}
else
{
callback(request.downloadHandler.text);
}
}
}
```
这个脚本创建了一个JavaInterface类,使用UnityWebRequest来发送HTTP GET和POST请求。在GetRequest和PostRequest函数中,使用UnityWebRequest创建一个HTTP请求,并设置请求头和请求体(如果有)。然后,使用SendWebRequest函数发送请求,并在请求完成后调用回调函数。
在Java中,创建一个接口,并使用以下代码:
```
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/{id}")
public String get(@PathVariable String id) {
// TODO: Handle GET request
return "Hello, Unity!";
}
@PostMapping("/post")
public String post(@RequestBody String body) {
// TODO: Handle POST request
return "Hello, Unity!";
}
}
```
这个接口使用Spring MVC框架来处理HTTP GET和POST请求。在get函数和post函数中,处理请求并返回一个字符串响应。
最后,在Unity中调用JavaInterface的GetRequest或PostRequest函数,发送HTTP GET或POST请求,并在请求完成后处理响应:
```
Dictionary<string, string> headers = new Dictionary<string, string>();
headers.Add("Content-Type", "application/json");
// 发送HTTP GET请求
JavaInterface java = new JavaInterface();
StartCoroutine(java.GetRequest("1", headers, (string response) => {
Debug.Log("Received response: " + response);
}));
// 发送HTTP POST请求
byte[] body = Encoding.UTF8.GetBytes("{\"name\": \"Unity\"}");
StartCoroutine(java.PostRequest("post", headers, body, (string response) => {
Debug.Log("Received response: " + response);
}));
```
在这个调用中,首先创建一个HTTP请求头,然后使用JavaInterface的GetRequest或PostRequest函数发送HTTP GET或POST请求,并在请求完成后处理响应。在这个示例中,响应将打印到控制台中。
阅读全文
相关推荐
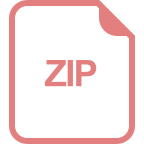















