c语言实现稀疏多项式相加问题创建一个新数组c:分别从头遍历比较a 和b的每一项;一个多项式已遍历完毕时,将另一个剩余项依次复制到c中。
时间: 2024-09-30 22:09:57 浏览: 36
在C语言中,实现稀疏多项式的相加问题,首先我们需要定义一个结构体来表示多项式的系数和指数,因为稀疏多项式通常包含大量的零元素。这里我们可以创建一个`sparse_poly`结构:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct sparse_poly {
int exponent; // 索引
long double coefficient; // 系数
} SparsePoly;
// 动态分配内存以存储结果
SparsePoly* addSparsePolys(SparsePoly *a, SparsePoly *b, int max_size) {
int i = 0, j = 0;
SparsePoly *c = malloc(max_size * sizeof(SparsePoly));
if (c == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
while (i < a->exponent && j < b->exponent) {
if (a->exponent > b->exponent) {
c[i++] = *a++;
} else if (a->exponent < b->exponent) {
c[i++] = *b++;
} else { // 如果指数相同
c[i].coefficient += a->coefficient + b->coefficient;
c[i++].exponent = a->exponent;
a++; b++;
}
}
// 将剩余的项添加到结果
while (i < a->exponent) {
c[i++] = *a++;
}
while (j < b->exponent) {
c[i++] = *b++;
}
// 清理多余的零项(如果有的话)
for (int k = 0; k < i && c[k].exponent == 0; k++) {
free(c + k);
c = realloc(c, (i - k) * sizeof(SparsePoly));
if (c == NULL) {
printf("Memory reallocation failed.\n");
return NULL;
}
}
c[i] = '\0'; // 表示结束
return c;
}
// 示例用法
void printSparsePoly(SparsePoly *poly) {
for (SparsePoly *p = poly; p->exponent != 0; p++) {
printf("%ldx^%d ", p->coefficient, p->exponent);
}
printf("\n");
}
int main() {
SparsePoly *a = {5, 2}, *b = {0, 3}, *c = addSparsePolys(a, b, 10); // 假设max_size足够大
printSparsePoly(c);
free(c); // 释放内存
return 0;
}
```
这个程序首先比较两个多项式的当前项,然后将较大的项添加到结果数组`c`中。当其中一个遍历完时,它会将另一个多项式的剩余项直接添加到结果。最后,我们还需要检查并清理结果数组中多余的零项。
阅读全文
相关推荐








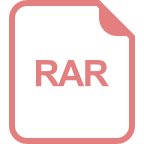







