1:用python以8数码问题为例实现A*算法的求解程序,设计估价函数
时间: 2023-12-31 22:04:44 浏览: 172
以下是基于Python的A*算法求解八数码问题的实现,同时设计了估价函数。
```python
import heapq
import numpy as np
# 定义目标状态
goal_state = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 0]])
# 定义状态转移函数
def move(state, direction):
new_state = state.copy()
x, y = np.where(state == 0)
if direction == 'up':
if x == 0:
return None
new_state[x, y], new_state[x-1, y] = new_state[x-1, y], new_state[x, y]
elif direction == 'down':
if x == 2:
return None
new_state[x, y], new_state[x+1, y] = new_state[x+1, y], new_state[x, y]
elif direction == 'left':
if y == 0:
return None
new_state[x, y], new_state[x, y-1] = new_state[x, y-1], new_state[x, y]
elif direction == 'right':
if y == 2:
return None
new_state[x, y], new_state[x, y+1] = new_state[x, y+1], new_state[x, y]
return new_state
# 定义估价函数
def heuristic(state):
count = 0
for i in range(3):
for j in range(3):
if state[i, j] != 0 and state[i, j] != goal_state[i, j]:
count += 1
return count
# 定义A*算法
def astar(start_state):
heap = [(heuristic(start_state), start_state, '', 0)]
closed = set()
while heap:
f, state, path, cost = heapq.heappop(heap)
if np.array_equal(state, goal_state):
return path
if str(state) in closed:
continue
closed.add(str(state))
for direction in ['up', 'down', 'left', 'right']:
new_state = move(state, direction)
if new_state is not None:
new_cost = cost + 1
new_path = path + direction
heapq.heappush(heap, (new_cost + heuristic(new_state), new_state, new_path, new_cost))
return None
# 测试
start_state = np.array([[2, 8, 3], [1, 6, 4], [7, 0, 5]])
path = astar(start_state)
if path is None:
print('无解')
else:
print('初始状态:')
print(start_state)
print('步骤:')
for direction in path:
start_state = move(start_state, direction)
print(start_state)
```
在上述代码中,`heuristic`函数采用了“曼哈顿距离”作为估价函数。曼哈顿距离是指两点在网格状平面上的距离之和,即:
$$h(i,j) = \sum_{i=1}^{3} \sum_{j=1}^{3} |state_{i,j} - goal_{i,j}|$$
其中,$state_{i,j}$表示当前状态第$i$行第$j$列的值,$goal_{i,j}$表示目标状态第$i$行第$j$列的值。
阅读全文
相关推荐
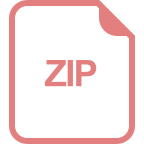
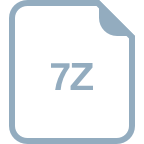






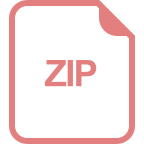
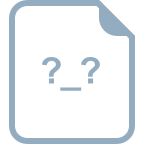




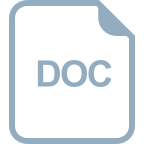


