编写一个Java程序,计算空间中任意两点之间的距离。程序要求首先定义一个接口IDistance,该接口定义了计算空间中任意两点之间距离的方法和显示一个显示空间任意点对象信息的默认方法,然后,定义三种类型的空间点类:二维空间点、三维空间点、四维空间点,这些类都要实现IDistance接口,最后,编写一个测试类,用于测试代码的正确性。
时间: 2023-05-15 08:06:55 浏览: 46
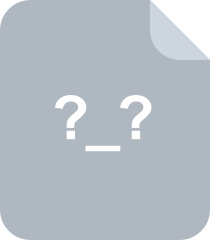
用java编写的求两点之间的距离

好的,以下是Java程序的代码:
// 定义计算空间中任意两点之间距离的接口
interface IDistance {
double distance(IDistance other);
void display();
}
// 定义二维空间点类
class Point2D implements IDistance {
private double x;
private double y;
public Point2D(double x, double y) {
this.x = x;
this.y = y;
}
public double distance(IDistance other) {
Point2D p = (Point2D) other;
return Math.sqrt(Math.pow(this.x - p.x, 2) + Math.pow(this.y - p.y, 2));
}
public void display() {
System.out.println("Point2D: (" + this.x + ", " + this.y + ")");
}
}
// 定义三维空间点类
class Point3D implements IDistance {
private double x;
private double y;
private double z;
public Point3D(double x, double y, double z) {
this.x = x;
this.y = y;
this.z = z;
}
public double distance(IDistance other) {
Point3D p = (Point3D) other;
return Math.sqrt(Math.pow(this.x - p.x, 2) + Math.pow(this.y - p.y, 2) + Math.pow(this.z - p.z, 2));
}
public void display() {
System.out.println("Point3D: (" + this.x + ", " + this.y + ", " + this.z + ")");
}
}
// 定义四维空间点类
class Point4D implements IDistance {
private double x;
private double y;
private double z;
private double w;
public Point4D(double x, double y, double z, double w) {
this.x = x;
this.y = y;
this.z = z;
this.w = w;
}
public double distance(IDistance other) {
Point4D p = (Point4D) other;
return Math.sqrt(Math.pow(this.x - p.x, 2) + Math.pow(this.y - p.y, 2) + Math.pow(this.z - p.z, 2) + Math.pow(this.w - p.w, 2));
}
public void display() {
System.out.println("Point4D: (" + this.x + ", " + this.y + ", " + this.z + ", " + this.w + ")");
}
}
// 测试类
public class TestDistance {
public static void main(String[] args) {
IDistance p1 = new Point2D(0, 0);
IDistance p2 = new Point2D(3, 4);
IDistance p3 = new Point3D(0, 0, 0);
IDistance p4 = new Point3D(1, 2, 3);
IDistance p5 = new Point4D(0, 0, 0, 0);
IDistance p6 = new Point4D(1, 2, 3, 4);
System.out.println("Distance between p1 and p2: " + p1.distance(p2));
System.out.println("Distance between p3 and p4: " + p3.distance(p4));
System.out.println("Distance between p5 and p6: " + p5.distance(p6));
p1.display();
p2.display();
p3.display();
p4.display();
p5.display();
p6.display();
}
}
阅读全文
相关推荐
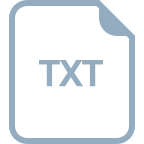

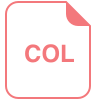
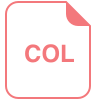
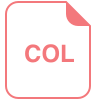
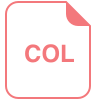
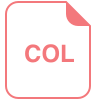
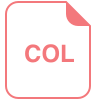
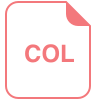
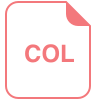
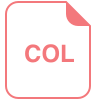
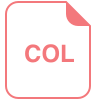
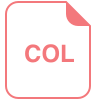
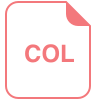
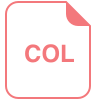
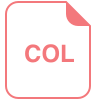