int clearFullRows(int gameArea[][COL]) { int count = 0; for (int i = ROW - 1; i >= 0; i--) { bool full = true; for (int j = 0; j < COL; j++) { if (gameArea[i][j] == 0) { full = false; break; } } if (full) { count++; for (int j = 0; j < COL; j++) { gameArea[i][j] = 0; gotoxy(j * MEASURE, i); setColor(0); cout << " "; } for (int k = i - 1; k >= 0; k--) { for (int j = 0; j < COL; j++) { gameArea[k + 1][j] = gameArea[k][j]; gotoxy(j * MEASURE, (k + 1)); setColor(gameArea[k + 1][j]); cout << "■"; } } i++; } } return count; } 消除此段代码的bug
时间: 2024-02-14 21:14:42 浏览: 25
这段代码的bug在于使用了goto语句,这是一种不推荐使用的控制语句,容易导致代码逻辑混乱和难以维护。建议使用其他方式代替goto语句。
以下是没有使用goto语句的修改后的代码:
```
int clearFullRows(int gameArea[][COL]) {
int count = 0;
for (int i = ROW - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < COL; j++) {
if (gameArea[i][j] == 0) {
full = false;
break;
}
}
if (full) {
count++;
for (int j = 0; j < COL; j++) {
gameArea[i][j] = 0;
gotoxy(j * MEASURE, i);
setColor(0);
cout << " ";
}
for (int k = i - 1; k >= 0; k--) {
for (int j = 0; j < COL; j++) {
gameArea[k + 1][j] = gameArea[k][j];
gotoxy(j * MEASURE, (k + 1));
setColor(gameArea[k + 1][j]);
cout << "■";
}
}
i++;
}
}
return count;
}
```
可以使用一个标志变量来记录是否需要更新行位置,然后在循环结束后再进行更新,如下所示:
```
int clearFullRows(int gameArea[][COL]) {
int count = 0;
bool needUpdate = false;
for (int i = ROW - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < COL; j++) {
if (gameArea[i][j] == 0) {
full = false;
break;
}
}
if (full) {
count++;
for (int j = 0; j < COL; j++) {
gameArea[i][j] = 0;
gotoxy(j * MEASURE, i);
setColor(0);
cout << " ";
}
needUpdate = true;
} else if (needUpdate) {
for (int j = 0; j < COL; j++) {
gameArea[i + count][j] = gameArea[i][j];
gotoxy(j * MEASURE, (i + count));
setColor(gameArea[i + count][j]);
cout << "■";
}
for (int j = 0; j < COL; j++) {
gameArea[i][j] = 0;
gotoxy(j * MEASURE, i);
setColor(0);
cout << " ";
}
}
}
return count;
}
```
这段代码使用了一个标志变量needUpdate,如果发现有满行需要消除,就将needUpdate设置为true。在循环中,如果发现当前行需要消除,就将needUpdate设置为true,否则就判断needUpdate是否为true,如果是,则将当前行向下移动count个单位。最后返回消除的行数。
相关推荐
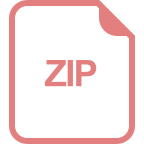
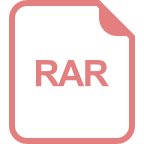
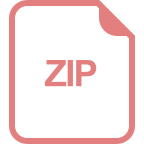














