请提供一段代码:判断自己的列表的维度
时间: 2023-08-31 07:36:56 浏览: 99
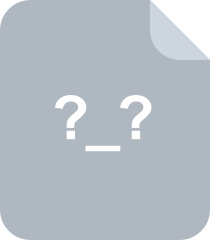
计算列表的度.py.py
### 回答1:
Sure, here's the code:
```python
import numpy as np
# Create a list
my_list = [[1, 2], [3, 4], [5, 6]]
# Convert list to numpy array
my_array = np.array(my_list)
# Get the dimensions of the array
dimensions = my_array.ndim
# Print the dimensions
print("The dimensions of my_array are:", dimensions)
```
This code will create a list `my_list`, then convert it to a numpy array `my_array`, and finally determine the dimensions of the array using the `ndim` attribute of numpy arrays.
### 回答2:
要判断一个列表的维度,可以使用递归算法来实现。下面是一个示例代码:
```python
def get_dimensions(lst):
if isinstance(lst, list):
return [len(lst)] + get_dimensions(lst[0])
else:
return []
# 示例测试用例
my_list = [1, 2, 3, 4]
print(get_dimensions(my_list)) # 输出:[4]
my_nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(get_dimensions(my_nested_list)) # 输出:[3, 3]
my_deeply_nested_list = [[[1], [2]], [[3], [4]], [[5], [6]]]
print(get_dimensions(my_deeply_nested_list)) # 输出:[3, 2, 1]
```
在这个代码中,`get_dimensions()` 函数将会递归地判断列表的维度。它首先判断输入的参数 `lst` 是否为列表类型,如果是,则返回一个由当前列表长度和递归调用列表的第一个元素得到的列表(即更深一层的维度)。如果输入参数不是列表类型,则返回一个空列表作为终止条件。
通过这个函数可以获取到列表的维度信息,并以列表的形式返回,如示例测试用例所示。
### 回答3:
可以使用Python的numpy库来判断列表的维度。下面是一个示例代码:
```python
import numpy as np
def get_dimension(arr):
dimension = np.array(arr).ndim
return dimension
# 测试代码
arr1 = [1, 2, 3, 4, 5]
arr2 = [[1, 2, 3], [4, 5, 6]]
arr3 = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]
print(get_dimension(arr1)) # 输出结果: 1
print(get_dimension(arr2)) # 输出结果: 2
print(get_dimension(arr3)) # 输出结果: 3
```
以上代码中,我们首先导入numpy库,并定义了一个函数`get_dimension`。该函数接受一个列表作为参数,并使用numpy库的`ndim`方法获取该列表的维度。最后,我们调用`get_dimension`函数来测试不同维度的列表。输出结果分别为1、2、3,分别对应列表的维度。
需要注意的是,为了使用numpy的ndim方法,需要先将列表转换为numpy数组。所以在代码中使用`np.array(arr)`来将列表`arr`转换为numpy数组。
阅读全文
相关推荐
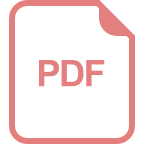
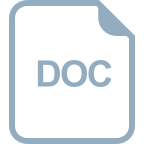

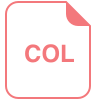
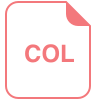
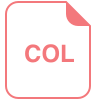












