将文件夹中的txt文件转为pcd格式
时间: 2024-01-26 13:03:55 浏览: 84
要将txt文件转换为pcd格式,需要使用点云处理软件,比如PCL(Point Cloud Library)。
具体步骤如下:
1. 安装PCL库,可以在官网上下载并按照说明进行安装。
2. 打开终端或命令行窗口,进入要转换的文件夹路径。
3. 执行以下命令将txt文件转换为pcd格式:
```
pcl_txt2pcd <input_file> <output_file>
```
其中,`<input_file>`是要转换的txt文件名,`<output_file>`是转换后的pcd文件名,可以自定义。
4. 执行命令后,等待转换完成即可。
需要注意的是,转换后的pcd文件可能需要进行后续处理和编辑,具体操作可以参考PCL库的相关文档和教程。
相关问题
写一个将文件夹中的txt文件转为pcd格式
以下是Python实现的将文件夹中的txt文件转换为pcd格式的代码示例:
```python
import os
import subprocess
def txt_to_pcd(txt_file, pcd_file):
"""
将单个txt文件转为pcd文件
"""
cmd = ['pcl_txt2pcd', txt_file, pcd_file]
p = subprocess.Popen(cmd, stdout=subprocess.PIPE)
p.wait()
def batch_txt_to_pcd(folder_path):
"""
批量将文件夹中的txt文件转为pcd文件
"""
# 创建输出文件夹
pcd_folder = os.path.join(folder_path, 'pcd_files')
if not os.path.exists(pcd_folder):
os.makedirs(pcd_folder)
# 遍历文件夹中的txt文件,逐个转换为pcd文件
for file_name in os.listdir(folder_path):
if file_name.endswith('.txt'):
txt_file = os.path.join(folder_path, file_name)
pcd_file = os.path.join(pcd_folder, file_name[:-4] + '.pcd')
txt_to_pcd(txt_file, pcd_file)
print(f'{txt_file} 转换为 {pcd_file} 完成!')
# 测试
folder_path = '/path/to/folder'
batch_txt_to_pcd(folder_path)
```
将上述代码保存为Python脚本,并将`/path/to/folder`替换为要转换的文件夹路径,运行即可批量将文件夹中的txt文件转换为pcd文件,并保存在与txt文件相同的文件夹中的`pcd_files`子文件夹中。
用c++写一个将文件夹中的txt文件转为pcd格式
以下是C++实现的将文件夹中的txt文件转换为pcd格式的代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <filesystem> // C++17标准的文件系统库,需要编译器支持
int txt_to_pcd(const std::string& txt_file, const std::string& pcd_file)
{
std::string cmd = "pcl_txt2pcd " + txt_file + " " + pcd_file;
int ret = std::system(cmd.c_str());
return ret;
}
void batch_txt_to_pcd(const std::string& folder_path)
{
// 创建输出文件夹
std::string pcd_folder = folder_path + "/pcd_files";
if (!std::filesystem::exists(pcd_folder)) {
std::filesystem::create_directory(pcd_folder);
}
// 遍历文件夹中的txt文件,逐个转换为pcd文件
for (const auto& entry : std::filesystem::directory_iterator(folder_path)) {
if (entry.path().extension() == ".txt") {
std::string txt_file = entry.path().string();
std::string pcd_file = pcd_folder + "/" + entry.path().stem().string() + ".pcd";
int ret = txt_to_pcd(txt_file, pcd_file);
if (ret == 0) {
std::cout << txt_file << " 转换为 " << pcd_file << " 完成!" << std::endl;
} else {
std::cerr << txt_file << " 转换失败!" << std::endl;
}
}
}
}
// 测试
int main()
{
std::string folder_path = "/path/to/folder";
batch_txt_to_pcd(folder_path);
return 0;
}
```
将上述代码保存为C++源代码文件,然后使用支持C++17标准的编译器编译并运行即可批量将文件夹中的txt文件转换为pcd文件,并保存在与txt文件相同的文件夹中的`pcd_files`子文件夹中。注意,需要将`/path/to/folder`替换为要转换的文件夹路径。
阅读全文
相关推荐








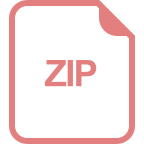
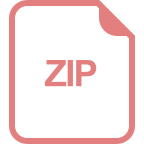



