如何使用链表结构构建二叉树,并实现其先序遍历的具体步骤和代码实现?
时间: 2024-10-24 20:16:37 浏览: 24
在使用链表结构构建二叉树时,首先需要理解每个节点通常包含两个指针,分别指向左子节点和右子节点。我们可以按照递归的方式将链表转换成二叉树:
1. **创建节点**:如果链表为空,则返回空节点。如果链表非空,可以创建一个新的二叉树节点,将其值设置为链表的第一个元素。
2. **分割链表**:将链表分为两部分,第一部分是剩余的节点,第二部分是剩余节点的前一个节点(作为新节点的右子节点)。这里需要用到两个临时变量保存头结点和新的右子节点。
3. **递归构建**:对剩下的链表递归地执行上述过程,构建出左子树。然后将新创建的二叉节点设置为原链表头节点的左子节点。
4. **先序遍历**:对于二叉树的先序遍历,顺序是根-左-右。所以遍历时,我们首先访问当前节点,然后递归遍历左子树,最后遍历右子树。
以下是使用Python实现的简单示例:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.left = None
self.right = None
def sortedListToBST(head):
if not head or not head.next:
return Node(head.data) if head else None
# 分割链表找到中间节点
slow, fast = head, head.next.next
while fast and fast.next:
slow = slow.next
fast = fast.next.next
mid = slow.next
slow.next = None
# 构建左、右子树并返回根节点
root = Node(mid.data)
root.left = sortedListToBST(head)
root.right = sortedListToBST(mid.next)
# 先序遍历
def preorder_traversal(root):
if root is not None:
print(root.data, end=" ")
preorder_traversal(root.left)
preorder_traversal(root.right)
# 示例
head = Node(4)
head.next = Node(2)
head.next.next = Node(6)
head.next.next.next = Node(1)
head.next.next.next.next = Node(3)
head.next.next.next.next.next = Node(5)
root = sortedListToBST(head)
preorder_traversal(root) # 输出: 4 2 6 1 3 5
```
阅读全文
相关推荐
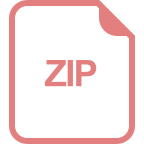
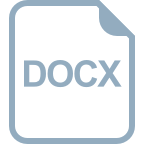
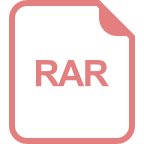

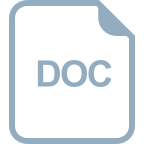

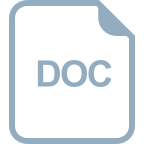






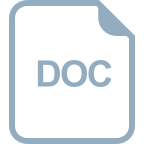
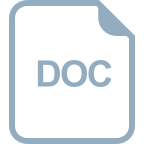



