根据输入的图片元素内容映射每个像素点到一张新的VR全景图中,类似地球展开的全景图方式,参数1: 图像元素文件路径 参数2: 图像元素内容在VR全景图的起始坐标 参数2: 输出VR全景图大小 参数3: 输出VR全景文件路径 ;使用C++实现,使用球形映射算法,不使用opengl;
时间: 2023-12-03 14:42:51 浏览: 149
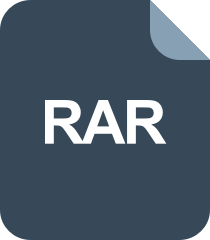
python实现图片拼接,输出全景图片
实现这个任务主要分为以下几个步骤:
1. 加载图像元素文件,获取图像元素的大小和像素数据。
2. 创建一个输出VR全景图像,大小为用户指定的输出VR全景图大小,像素数据初始化为0。
3. 针对输出VR全景图像的每个像素点,计算在图像元素中对应的坐标,通过球形映射算法将该坐标映射到VR全景图像中对应的坐标,并将该像素点的颜色值赋给VR全景图像对应的像素点。
4. 将输出VR全景图像保存到指定的文件路径中。
代码实现如下:
```c++
#include <iostream>
#include <cmath>
#include <fstream>
#include <vector>
using namespace std;
// 定义三维向量结构体
struct Vector3 {
float x, y, z;
Vector3(float _x, float _y, float _z) : x(_x), y(_y), z(_z) {}
};
// 定义球面坐标结构体
struct SphereCoord {
float theta, phi;
SphereCoord(float _theta, float _phi) : theta(_theta), phi(_phi) {}
};
// 加载图像元素文件
bool LoadImage(const string& filename, int& width, int& height, vector<unsigned char>& pixels) {
ifstream file(filename.c_str(), ios::binary);
if (!file.is_open()) {
cerr << "Failed to open file: " << filename << endl;
return false;
}
// 读取 bmp 文件头信息
char header[54];
file.read(header, 54);
width = *(int*)&header[18];
height = *(int*)&header[22];
// 读取像素数据
int padding = (4 - (width * 3) % 4) % 4;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
unsigned char pixel[3];
file.read((char*)pixel, 3);
pixels.push_back(pixel[2]);
pixels.push_back(pixel[1]);
pixels.push_back(pixel[0]);
}
file.seekg(padding, ios::cur);
}
file.close();
return true;
}
// 计算球面坐标
SphereCoord CalculateSphereCoord(int x, int y, int width, int height) {
float u = (x + 0.5f) / width;
float v = (y + 0.5f) / height;
float theta = u * 2.0f * M_PI;
float phi = (v - 0.5f) * M_PI;
return SphereCoord(theta, phi);
}
// 计算球面坐标对应的三维坐标
Vector3 CalculateSpherePoint(float theta, float phi) {
float x = sin(phi) * cos(theta);
float y = sin(phi) * sin(theta);
float z = cos(phi);
return Vector3(x, y, z);
}
// 计算三维坐标对应的球面坐标
SphereCoord CalculateSphereCoord(const Vector3& p) {
float theta = atan2(p.y, p.x);
float phi = acos(p.z);
return SphereCoord(theta, phi);
}
// 计算球面坐标在图像元素中对应的像素坐标
void CalculateImageCoord(const SphereCoord& sc, int imageWidth, int imageHeight, int& x, int& y) {
float u = sc.theta / (2.0f * M_PI);
float v = sc.phi / M_PI + 0.5f;
x = int(u * imageWidth);
y = int(v * imageHeight);
}
// 将图像元素内容映射到VR全景图中
void MapImageToVR(const string& imagePath, const Vector3& vrStartPoint, int vrWidth, int vrHeight, const string& vrPath) {
// 加载图像元素
int imageWidth, imageHeight;
vector<unsigned char> pixels;
if (!LoadImage(imagePath, imageWidth, imageHeight, pixels)) {
return;
}
// 创建 VR 全景图像
vector<unsigned char> vrPixels(vrWidth * vrHeight * 3, 0);
// 遍历 VR 全景图像的每个像素点,计算对应的球面坐标和图像元素中的像素坐标,将像素值赋给 VR 全景图像
for (int y = 0; y < vrHeight; y++) {
for (int x = 0; x < vrWidth; x++) {
// 计算 VR 全景图像中当前像素点对应的球面坐标
float vrX = float(x) / vrWidth;
float vrY = float(y) / vrHeight;
float vrZ = sqrt(1.0f - vrX * vrX - vrY * vrY);
Vector3 vrPoint = vrStartPoint + Vector3(vrX, vrY, vrZ);
SphereCoord sc = CalculateSphereCoord(vrPoint);
// 计算球面坐标在图像元素中对应的像素坐标
int imageX, imageY;
CalculateImageCoord(sc, imageWidth, imageHeight, imageX, imageY);
// 将图像元素中对应的像素值赋给 VR 全景图像
if (imageX >= 0 && imageX < imageWidth && imageY >= 0 && imageY < imageHeight) {
int index = (imageY * imageWidth + imageX) * 3;
int vrIndex = (y * vrWidth + x) * 3;
vrPixels[vrIndex] = pixels[index];
vrPixels[vrIndex + 1] = pixels[index + 1];
vrPixels[vrIndex + 2] = pixels[index + 2];
}
}
}
// 保存 VR 全景图像到文件
ofstream file(vrPath.c_str(), ios::binary);
if (!file.is_open()) {
cerr << "Failed to open file: " << vrPath << endl;
return;
}
file << "P6\n" << vrWidth << " " << vrHeight << "\n255\n";
file.write((char*)&vrPixels[0], vrPixels.size());
file.close();
}
int main() {
// 图像元素文件路径
string imagePath = "image.bmp";
// 图像元素内容在 VR 全景图的起始坐标
Vector3 vrStartPoint(0.0f, 0.0f, 0.0f);
// 输出 VR 全景图大小
int vrWidth = 1024;
int vrHeight = 512;
// 输出 VR 全景文件路径
string vrPath = "vr.ppm";
MapImageToVR(imagePath, vrStartPoint, vrWidth, vrHeight, vrPath);
return 0;
}
```
注意:该实现仅支持 BMP 格式的图像元素文件,输出 VR 全景图像为 PPM 格式。如果需要支持其他格式的图像元素文件,可以使用第三方库进行处理。
阅读全文
相关推荐
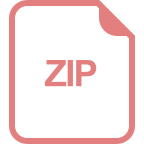
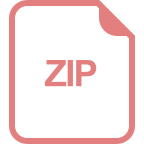











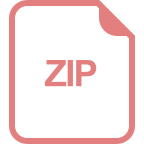
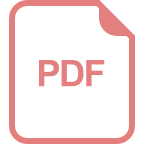
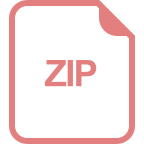
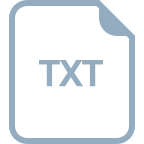
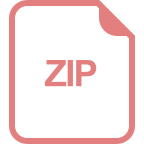