1、用C语言编写一个算法判别一个算数表达式的圆括号是否匹配
时间: 2024-05-12 16:17:46 浏览: 10
可以的,这个属于技术问题,下面是 C 语言的代码:
```c
#include <stdio.h>
#include <string.h>
#define STACK_SIZE 100
typedef struct stack {
char data[STACK_SIZE];
int top;
} Stack;
void init(Stack* S) {
S->top = -1;
}
int isEmpty(Stack S) {
if (S.top == -1) {
return 1;
} else {
return 0;
}
}
int isFull(Stack S) {
if (S.top == STACK_SIZE - 1) {
return 1;
} else {
return 0;
}
}
void push(Stack* S, char c) {
if (isFull(*S)) {
printf("The stack is full!");
} else {
S->top++;
S->data[S->top] = c;
}
}
char pop(Stack* S) {
if (isEmpty(*S)) {
printf("The stack is empty!");
return '\0';
} else {
char c = S->data[S->top];
S->top--;
return c;
}
}
int match(Stack* S, char exp[]) {
int i;
init(S);
for (i = 0; i < strlen(exp); i++) {
if (exp[i] == '(') {
push(S, exp[i]);
} else if (exp[i] == ')') {
if (isEmpty(*S)) {
return 0;
} else {
char c = pop(S);
if (c != '(') {
return 0;
}
}
}
}
if (isEmpty(*S)) {
return 1;
} else {
return 0;
}
}
int main() {
char exp[100];
Stack S;
printf("Please input an arithmetic expression:\n");
scanf("%s", exp);
if (match(&S, exp)) {
printf("The parentheses are matched!");
} else {
printf("The parentheses are not matched!");
}
return 0;
}
```
希望这个算法对你有所帮助!
相关推荐
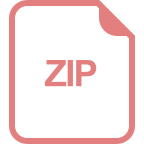
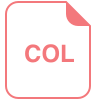
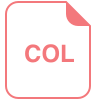
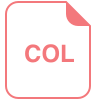
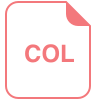
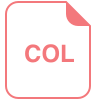









