写出基于树莓派csi摄像头实时仓库谷物计数和仓库温湿度和烟雾检测系统的代码
时间: 2023-06-20 08:04:51 浏览: 87
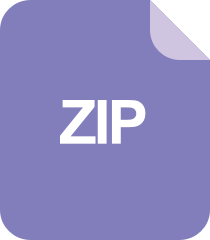
基于树莓派室内温湿度监控服务的+源代码+文档说明
抱歉,我无法直接为您提供完整的代码。但是,我可以为您提供一些基本的思路和代码片段,以帮助您开始编写这个系统。
1. 首先,您需要安装CSI摄像头,并安装相关的驱动程序和Python库。您可以使用以下命令安装所需的库:
```bash
sudo apt-get update
sudo apt-get install python-picamera
sudo apt-get install python3-picamera
```
2. 接下来,您需要使用OpenCV库来处理图像并进行计数。以下是一些基本的代码片段,可以帮助您开始:
```python
import cv2
# Initialize camera
camera = cv2.VideoCapture(0)
# Define kernel for image processing
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
while True:
# Capture frame-by-frame
ret, frame = camera.read()
# Convert to grayscale
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Perform image processing
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)[1]
thresh = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel)
# Find contours and draw bounding boxes
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
# Display the resulting frame
cv2.imshow('frame', frame)
# Exit program if 'q' is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the camera and close all windows
camera.release()
cv2.destroyAllWindows()
```
3. 接下来,您需要使用DHT11传感器来检测仓库的温度和湿度。以下是一些基本的代码片段,可以帮助您开始:
```python
import Adafruit_DHT
# Define sensor type and pin number
sensor = Adafruit_DHT.DHT11
pin = 4
# Read temperature and humidity
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
# Print values to console
print('Temperature: {0:0.1f} C'.format(temperature))
print('Humidity: {0:0.1f} %'.format(humidity))
```
4. 最后,您需要使用MQ-2传感器来检测烟雾。以下是一些基本的代码片段,可以帮助您开始:
```python
import RPi.GPIO as GPIO
import time
# Define pin number for MQ-2 sensor
pin = 17
# Set up GPIO
GPIO.setmode(GPIO.BCM)
GPIO.setup(pin, GPIO.IN)
while True:
# Read sensor value
sensor_value = GPIO.input(pin)
# Print value to console
if sensor_value:
print('Smoke detected!')
else:
print('No smoke detected.')
# Wait for a short time before reading again
time.sleep(0.5)
```
这些代码片段只是给您提供了一个起点,您需要根据自己的具体需求进行修改和完善。同时,确保您已经正确地连接了所有传感器,并且您的代码可以正确地读取它们的值。
阅读全文
相关推荐
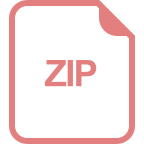
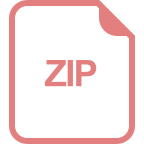
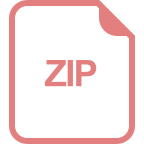
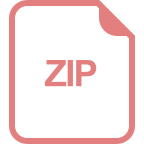
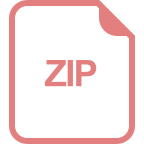
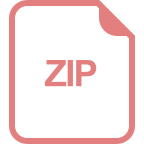
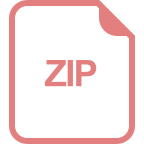
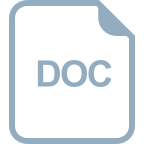
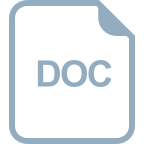








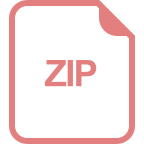