module servo( input clk, //时钟 50MHz input sw1, //调速按键 output pwm //pwm输出 ); reg [19:0] pwm_val; //占空比计数值 reg [3:0] speed = 4'd2; //转向角度选择 reg pwm; parameter max_cnt=1000_000; reg [19:0] clk_cnt; always @(posedge clk)begin //产生20ms周期计时 if(clk_cnt==max_cnt)begin clk_cnt=20'b0; end else begin clk_cnt=clk_cnt+1'b1; end end //PWM产生模块 always @ (posedge clk) begin if(clk_cnt < pwm_val) begin //如果在pwm_val内,输出高电平 pwm <=1'b1; end else begin //如果超出pwm_val,则输出低电平 pwm <=1'b0; //输出低电平 end case(speed) 1: pwm_val <= 20'd50_000; //占空比为5% 1ms 2: pwm_val <= 20'd100_000; //10% 2ms 3: pwm_val <= 20'd25_000; 4: pwm_val <= 20'd75_000; 5: pwm_val <= 20'd1_000; default: pwm_val <= 20'd1_000; endcase end //开关调速 always @ (posedge clk) begin if(sw1) begin speed <= 4'd1; end else begin speed <= 4'd2; end end endmodule
时间: 2023-06-19 19:03:18 浏览: 64
这是一个 Verilog HDL 语言编写的舵机控制模块,主要实现舵机的转向角度和速度控制。其中包括一个时钟模块和一个 PWM 产生模块。
时钟模块使用了一个计数器来产生一个 20ms 的周期计时信号。PWM 产生模块通过比较计数器的值和占空比计数值,来产生 PWM 信号控制舵机的转向角度和速度。开关调速模块通过控制转向角度选择信号,来实现舵机的转向角度调整。
相关问题
com/netflix/servo/monitor/Monitors
com/netflix/servo/monitor/Monitors是一个Java类的路径。根据引用\[3\]中的错误信息,这个类没有被找到,导致了ClassNotFoundException异常。这个错误通常是由于缺少相关的依赖库或者类路径配置错误引起的。根据引用\[2\]中的信息,可以通过在pom.xml文件中添加com.netflix.servo:servo-core:0.12.7依赖来解决这个问题。这个依赖库包含了com.netflix.servo.monitor.Monitors类,添加后重新编译和运行程序应该就可以找到这个类了。
#### 引用[.reference_title]
- *1* [servo 监控](https://blog.csdn.net/xuanmuzhao/article/details/80155367)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [spring cloud报错解决:java.lang.ClassNotFoundException: com.netflix.servo.monitor.Monitors](https://blog.csdn.net/weixin_33913377/article/details/85918439)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
servo myservo; //舵机
这是 Arduino 代码中定义一个名为 `myservo` 的舵机对象,用于控制舵机的旋转角度。通常在设置舵机之前需要先指定舵机控制引脚,例如:
```c++
servo myservo; //定义舵机对象
int servoPin = 9; //定义舵机控制引脚
myservo.attach(servoPin); //将舵机对象与控制引脚绑定
```
之后可以通过 `myservo.write()` 方法来控制舵机旋转到指定的角度。例如:
```c++
myservo.write(90); //将舵机旋转到 90 度
```
相关推荐
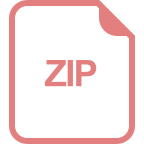












