设计一个有向图和一个无向图,任选一种存储结构,完成有向图和无向图的 DFS(深 度优先遍历)和BFS(广度优先遍历)的操作c语言。
时间: 2024-12-16 17:22:08 浏览: 6
设计一个有向图和无向图的数据结构,我们可以选择邻接矩阵、邻接表或者边集作为存储结构。这里我们以邻接表为例,它更适合表示稀疏图,即边的数量远小于顶点数的平方。
首先,我们需要定义节点和图的基本结构:
```c
// 定义节点结构
typedef struct Node {
int value; // 节点值
struct Node* next; // 指向相邻节点的指针
} Node;
// 定义无向图和有向图的结构体
typedef struct Graph {
int numVertices; // 顶点数
Node** adjList; // 邻接列表
} Graph;
```
对于DFS(深度优先搜索)操作:
```c
void dfs(Graph* graph, int vertex, int visited[]) {
visited[vertex] = 1;
printf("%d ", vertex);
Node* temp = graph->adjList[vertex];
while (temp != NULL) {
if (!visited[temp->value]) {
dfs(graph, temp->value, visited);
}
temp = temp->next;
}
}
```
对于BFS(广度优先搜索)操作:
```c
void bfs(Graph* graph, int start) {
int queue[graph->numVertices], front = 0, rear = 0;
for (int i = 0; i < graph->numVertices; ++i) {
visited[i] = 0;
}
visited[start] = 1;
queue[rear++] = start;
while (front < rear) {
int vertex = queue[front++];
printf("%d ", vertex);
for (Node* temp = graph->adjList[vertex]; temp != NULL; temp = temp->next) {
if (!visited[temp->value]) {
visited[temp->value] = 1;
queue[rear++] = temp->value;
}
}
}
}
```
阅读全文
相关推荐
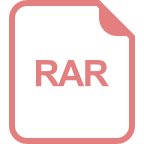
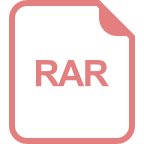
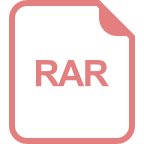
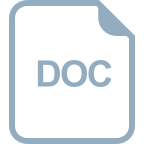
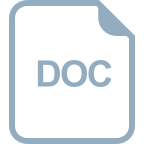
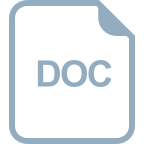
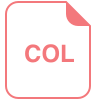










