用分治法解决凸包问题有可视化c语言
时间: 2024-05-04 22:21:01 浏览: 115
以下是使用C语言实现分治法解决凸包问题的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <graphics.h>
typedef struct point {
int x;
int y;
} Point;
typedef struct line {
Point p1;
Point p2;
} Line;
void drawLine(Line l) {
line(l.p1.x, l.p1.y, l.p2.x, l.p2.y);
}
float distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
float crossProduct(Point p1, Point p2, Point p3) {
return (p2.x - p1.x) * (p3.y - p1.y) - (p3.x - p1.x) * (p2.y - p1.y);
}
void drawConvexHull(Point *points, int n) {
if (n <= 1) return;
Point leftmost = points[0];
Point rightmost = points[0];
for (int i = 1; i < n; i++) {
if (points[i].x < leftmost.x) {
leftmost = points[i];
}
if (points[i].x > rightmost.x) {
rightmost = points[i];
}
}
setcolor(RED);
drawLine((Line) {leftmost, rightmost});
Point *leftPoints = (Point *) malloc((n + 1) * sizeof(Point));
Point *rightPoints = (Point *) malloc((n + 1) * sizeof(Point));
int leftCount = 0;
int rightCount = 0;
for (int i = 0; i < n; i++) {
if (crossProduct(leftmost, rightmost, points[i]) > 0) {
leftPoints[leftCount++] = points[i];
} else if (crossProduct(leftmost, rightmost, points[i]) < 0) {
rightPoints[rightCount++] = points[i];
}
}
drawConvexHull(leftPoints, leftCount);
drawConvexHull(rightPoints, rightCount);
free(leftPoints);
free(rightPoints);
}
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
Point points[] = {{50, 50}, {100, 100}, {150, 50}, {200, 200}, {250, 50}, {300, 100}, {350, 50}, {400, 150}};
int n = sizeof(points) / sizeof(points[0]);
drawConvexHull(points, n);
getch();
closegraph();
return 0;
}
```
这段代码使用了图形库来进行可视化,可以在运行程序时看到凸包的绘制过程。具体实现思路是使用分治法将点集分为左右两部分,然后递归地求解左右两部分的凸包,最后将左右两个凸包合并成一个凸包。在合并时,找到左边凸包的最右边的点和右边凸包的最左边的点,然后连接这两个点,将它们之间的线段作为凸包的边。
阅读全文
相关推荐
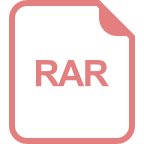
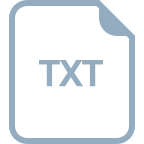

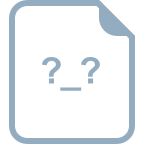


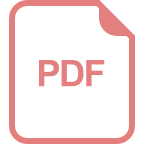
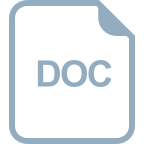
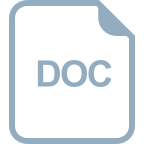
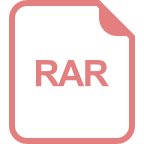
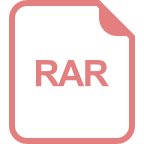
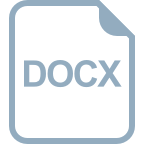




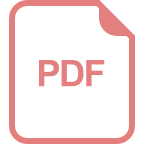