求n个数的最小公因数,用c语言
时间: 2024-10-14 21:12:58 浏览: 18
求几个数的最小公因数(Least Common Multiple, LCM)在C语言中通常通过欧几里得算法(Euclidean algorithm)或辗转相除法找到最大公约数(Greatest Common Divisor, GCD),然后用乘积除以GCD来得到LCM。下面是一个简单的示例,演示如何计算两个数的GCD,进而推导出多个数的LCM:
首先,我们需要一个函数来计算GCD:
```c
// Euclidean algorithm for finding GCD of two numbers
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
// Function to find LCM using GCD
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
```
对于多个数的情况,我们可以先计算前两个数的LCM,然后再用这个结果和下一个数计算新的LCM,直到所有的数都被处理过。这里有一个递归版本的例子:
```c
// Function to find LCM of an array
int find_lcm(int arr[], int n) {
int first = arr[0], i, temp;
for (i = 1; i < n; i++) {
temp = lcm(first, arr[i]);
first = temp;
}
return first;
}
```
你可以像这样使用上述`find_lcm`函数来求一组整数的最小公因数:
```c
#include <stdio.h>
int main() {
int num[] = {5, 7, 15};
int n = sizeof(num) / sizeof(num[0]);
int result = find_lcm(num, n);
printf("The least common multiple of the numbers is: %d\n", result);
return 0;
}
阅读全文
相关推荐
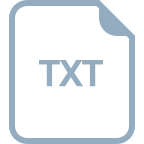
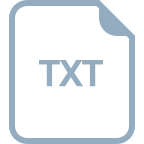
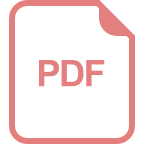















