用cpp建立一个合适的继承体系shape类,测试 public 继承下的基类不同访问权限的派生类调用,仅需简单测试
时间: 2024-10-17 07:14:32 浏览: 7
在 C++ 中,我们可以创建一个简单的形状继承体系,其中 `Shape` 类作为基类,然后定义一些公有、私有和保护的派生类。这里是一个示例:
```cpp
#include <iostream>
// 基类 Shape
class Shape {
public:
// 公共成员函数(默认权限)
virtual double getArea() const = 0; // 定义纯虚函数,表示所有派生类都需要覆盖此方法
protected:
// 保护成员变量
int shapeType;
private:
// 私有成员函数和数据
Shape(int type) : shapeType(type) {}
};
// 公开继承的派生类 Circle
class Circle : public Shape {
public:
explicit Circle(double radius) : Shape(CIRCLE), radius_(radius) {} // 使用 CIRCLE 常量作为类型标识
// 公有访问权限的 area() 函数
double getArea() const override { return M_PI * radius_ * radius_; }
private:
const double radius_;
};
// 公开继承的派生类 Rectangle
class Rectangle : public Shape {
public:
Rectangle(double width, double height) : Shape(RECTANGLE), width_(width), height_(height) {}
// 公有访问权限的 getArea() 函数
double getArea() const override { return width_ * height_; }
private:
const double width_, height_;
};
int main() {
Circle circle(5);
std::cout << "Circle Area: " << circle.getArea() << std::endl;
Rectangle rectangle(4, 6);
std::cout << "Rectangle Area: " << rectangle.getArea() << std::endl;
return 0;
}
```
在这个例子中,我们有三个部分:
1. `Shape` 类:这是一个抽象基类,它有一个纯虚函数 `getArea()`,用于计算形状面积。`shapeType` 是一个保护成员变量。
2. `Circle` 和 `Rectangle` 类:它们都是公开继承自 `Shape` 的派生类,并分别实现了 `getArea()` 函数。`Circle` 的 `radius_` 是私有的,而 `Rectangle` 的 `width_` 和 `height_` 也是私有的。这两个派生类都通过公共的构造函数设置了各自类的属性。
3. `main()` 函数:在这里,我们创建了 `Circle` 和 `Rectangle` 的实例,并调用了它们的 `getArea()` 函数,展示了如何在基类的公共继承下,通过派生类的不同访问权限调用函数。
阅读全文
相关推荐
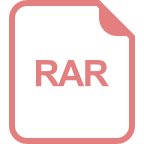
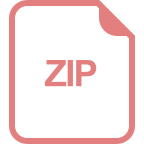
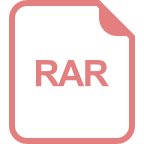















