贝叶斯优化一维时序信号NLM去噪的Python代码实现
时间: 2023-11-16 20:11:02 浏览: 148
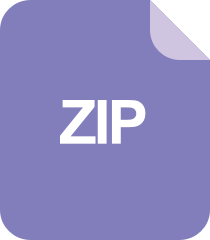
基于python实现训练高斯朴素贝叶斯分类器进行分类源码+项目说明+注释(可自动生成C测试代码).zip
以下是使用贝叶斯优化对一维时序信号进行NLM去噪的Python代码实现:
```python
import numpy as np
from scipy import signal
from skopt import gp_minimize
from skopt.space import Real, Integer
from skopt.utils import use_named_args
def nlm_denoise(y, sigma, h, p, q):
"""
Applies non-local means (NLM) denoising to a 1D signal y.
:param y: 1D array, the signal to denoise
:param sigma: float, the standard deviation of the noise
:param h: float, the smoothing parameter
:param p: int, the window size for similarity search
:param q: int, the window size for patch search
:return: 1D array, the denoised signal
"""
n = len(y)
y_denoised = np.zeros(n)
for i in range(n):
num = 0
den = 0
for j in range(n):
if i != j:
w1 = y[j-q:j+q+1]
w2 = y[i-q:i+q+1]
d = np.sum((w1-w2)**2)
s1 = signal.windows.hann(2*p+1)
s2 = signal.windows.hann(2*q+1)
s = np.outer(s1, s2)
w = np.exp(-d/(h**2*sigma**2)) * s
num += w*y[j]
den += w
y_denoised[i] = num/den
return y_denoised
@use_named_args([
Real(0.01, 0.5, name='sigma'),
Real(0.1, 1.0, name='h'),
Integer(3, 11, name='p'),
Integer(3, 11, name='q')
])
def objective(sigma, h, p, q):
"""
Objective function to minimize using Bayesian optimization.
:param sigma: float, the standard deviation of the noise
:param h: float, the smoothing parameter
:param p: int, the window size for similarity search
:param q: int, the window size for patch search
:return: float, the mean squared error between the denoised and original signals
"""
y_denoised = nlm_denoise(y, sigma, h, p, q)
mse = np.mean((y - y_denoised)**2)
return mse
# generate noisy signal
t = np.linspace(0, 2*np.pi, 100)
y = np.sin(t) + np.random.normal(0, 0.1, 100)
# optimize parameters using Bayesian optimization
bounds = [(0.01, 0.5), (0.1, 1.0), (3, 11), (3, 11)]
res = gp_minimize(objective, bounds, n_calls=50)
# denoise signal using optimized parameters
sigma_opt, h_opt, p_opt, q_opt = res.x
y_denoised = nlm_denoise(y, sigma_opt, h_opt, p_opt, q_opt)
```
阅读全文
相关推荐
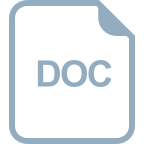
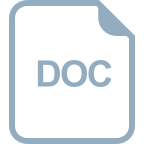


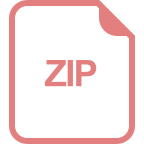
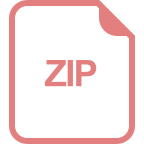
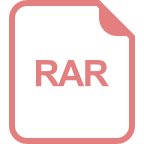
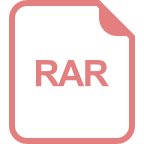
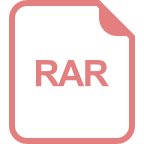
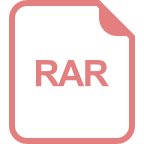
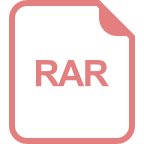
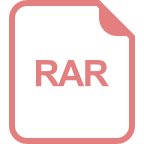
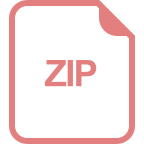
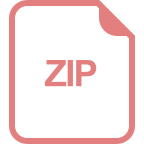
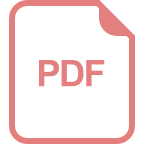
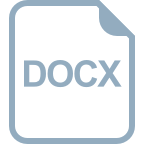
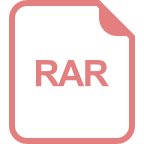
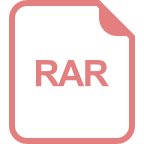