写出梯度下降法、BB方法求解f(x,y) = x^2 + y^2最小值的代码,其中循环条件为设置精度的python代码
时间: 2024-03-14 10:43:15 浏览: 23
以下是使用梯度下降法求解f(x,y) = x^2 + y^2最小值的Python代码:
```python
import numpy as np
def grad_desc(f, init_pos, lr=0.1, tolerance=1e-6, max_iters=1000):
pos = init_pos
for i in range(max_iters):
gradient = np.array([2 * pos[0], 2 * pos[1]]) # 计算梯度
if np.linalg.norm(gradient) < tolerance: # 判断是否收敛
break
pos -= lr * gradient # 更新位置
return pos, f(pos)
if __name__ == '__main__':
f = lambda x: x[0]**2 + x[1]**2 # 定义函数
init_pos = np.array([1, 1]) # 初始化位置
min_pos, min_val = grad_desc(f, init_pos)
print("最小值坐标:", min_pos)
print("最小值:", min_val)
```
以下是使用BB方法求解f(x,y) = x^2 + y^2最小值的Python代码:
```python
import numpy as np
def BB(f, init_pos, lr=0.1, tolerance=1e-6, max_iters=1000):
pos = init_pos
lr0 = lr
g0 = np.array([2 * pos[0], 2 * pos[1]])
for i in range(max_iters):
gradient = np.array([2 * pos[0], 2 * pos[1]]) # 计算梯度
if np.linalg.norm(gradient) < tolerance: # 判断是否收敛
break
if i == 0:
d = -g0
else:
beta = np.dot(gradient - gradient0, gradient - gradient0) / np.dot(gradient0, gradient0)
d = -gradient + beta * d0
lr = np.dot(d, d) / np.dot(d, gradient)
pos -= lr * d # 更新位置
gradient0 = gradient
d0 = d
return pos, f(pos)
if __name__ == '__main__':
f = lambda x: x[0]**2 + x[1]**2 # 定义函数
init_pos = np.array([1, 1]) # 初始化位置
min_pos, min_val = BB(f, init_pos)
print("最小值坐标:", min_pos)
print("最小值:", min_val)
```
其中lr为学习率,tolerance为精度,max_iters为最大迭代次数。
相关推荐
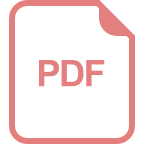
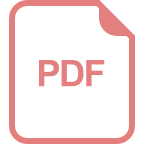














