创建一个矩阵类,写出:1.构造函数;2.重载运算符<<;3.输入矩阵数据的input函数
时间: 2024-12-06 09:23:59 浏览: 8
创建一个矩阵类(Matrix),我们可以按照以下步骤设计:
1. 构造函数(Constructor):
```cpp
class Matrix {
public:
// 构造函数,初始化矩阵的行数和列数为0,元素为默认值
Matrix(int rows = 0, int cols = 0) : m_rows(rows), m_cols(cols) {
data.resize(rows, std::vector<double>(cols, 0));
}
private:
int m_rows;
int m_cols;
std::vector<std::vector<double>> data;
};
```
这里假设我们使用`double`作为矩阵的元素类型。
2. 重载运算符<<(Output operator):
这个操作符用于将矩阵内容输出到控制台,方便查看。
```cpp
std::ostream& operator<<(std::ostream& out, const Matrix& matrix) {
out << "Matrix:\n";
for (int i = 0; i < matrix.m_rows; ++i) {
for (int j = 0; j < matrix.m_cols; ++j) {
out << matrix.data[i][j] << ' ';
}
out << '\n';
}
return out;
}
```
3. 输入矩阵数据的input函数:
```cpp
void input(const std::string& filename, Matrix& matrix) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open file");
}
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
int rowSize;
if (!(iss >> rowSize) || rowSize != matrix.m_cols) {
throw std::runtime_error("Invalid matrix format");
}
double value;
for (int j = 0; j < rowSize; ++j) {
if (!(iss >> value)) {
throw std::runtime_error("Invalid value in matrix");
}
matrix.data.push_back({value});
}
}
file.close();
}
```
这个函数从文件中读取矩阵数据并存储到`Matrix`对象中,如果文件格式错误则抛出异常。
阅读全文
相关推荐
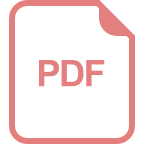
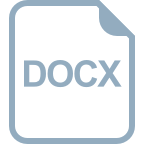
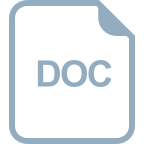

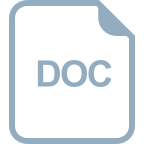
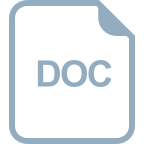
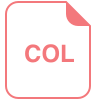











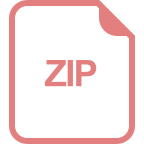