运用Java代码和dfaRecognize函数实现E' → E → E + T → E + F → E + id → id * id + id
时间: 2024-01-24 18:01:45 浏览: 62
首先,我们需要定义一个 DFA(Deterministic Finite Automaton)来识别该文法。下面是 DFA 的状态转移图:
```
+-----------+
| |
| start |
| |
+-----+-----+
|
|
v
+-----+-----+
| |
| E |
| |
+-----+-----+
|
|
+-----------+-----------+
| |
v v
+-----+-----+ +-----+-----+
| | | |
| T | | F |
| | | |
+-----+-----+ +-----+-----+
| |
| |
v v
+-----+-----+ +-----+-----+
| | | |
| id | | id |
| | | |
+-----------+ +-----------+
| |
| |
v v
+-----+-----+ +-----+-----+
| | | |
| + | | * |
| | | |
+-----------+ +-----------+
| |
| |
v v
+-----+-----+ +-----+-----+
| | | |
| E | | id |
| | | |
+-----------+ +-----------+
```
其中,状态 E、T、F 分别表示对应的非终结符,状态 id、+、* 表示对应的终结符。
根据 DFA 的状态转移图,我们可以用 Java 代码实现一个 DFA 识别器。下面是示例代码:
```java
public class DfaRecognize {
private static final int STATE_START = 0;
private static final int STATE_E = 1;
private static final int STATE_T = 2;
private static final int STATE_F = 3;
private static final int STATE_ID = 4;
private static final int STATE_PLUS = 5;
private static final int STATE_MULTIPLY = 6;
private static final int[] FINAL_STATES = {STATE_ID, STATE_PLUS, STATE_MULTIPLY};
private static final int[][] TRANS_TABLE = {
// id + * (other)
/* start */{STATE_ID, -1, -1, STATE_E},
/* E */{STATE_T, STATE_PLUS, -1, -1},
/* T */{STATE_F, -1, STATE_MULTIPLY, -1},
/* F */{STATE_ID, -1, -1, -1},
/* id */{-1, -1, -1, -1},
/* + */{-1, -1, -1, STATE_E},
/* * */{STATE_ID, -1, -1, -1},
};
public static boolean recognize(String input) {
int state = STATE_START;
for (char ch : input.toCharArray()) {
int inputType;
if (ch == 'id') {
inputType = 0;
} else if (ch == '+') {
inputType = 1;
} else if (ch == '*') {
inputType = 2;
} else {
inputType = 3;
}
state = TRANS_TABLE[state][inputType];
if (state == -1) {
return false;
}
}
for (int finalState : FINAL_STATES) {
if (state == finalState) {
return true;
}
}
return false;
}
}
```
最后,我们可以使用 DFA 识别器来判断一个字符串是否符合该文法:
```java
String input = "id * id + id";
if (DfaRecognize.recognize(input)) {
System.out.println("符合文法");
} else {
System.out.println("不符合文法");
}
```
阅读全文
相关推荐
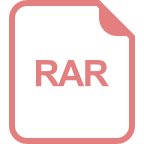
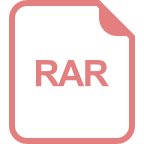














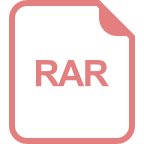