在unity中,我有5个对象,A,B,C,D,E,他们身上都有对应的Animator以及动画,请你帮我写一个脚本,通过按钮用来控制他们的动画播放,暂停与重新播放
时间: 2024-09-13 16:03:44 浏览: 37
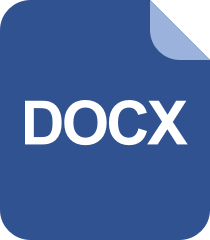
Unity3D中多个动画同时存在时间的控制代码1
在Unity中,你可以通过编写一个C#脚本来实现按钮控制不同对象的动画播放、暂停和重新播放。下面是一个简单的示例脚本,你可以根据自己的具体需求进行调整:
```csharp
using UnityEngine;
public class AnimationController : MonoBehaviour
{
private Animator[] animators; // 存储所有Animator组件的数组
private int playIndex = 0; // 当前播放动画的对象索引
void Start()
{
// 初始化时获取所有Animator组件
animators = new Animator[] { A.GetComponent<Animator>(), B.GetComponent<Animator>(),
C.GetComponent<Animator>(), D.GetComponent<Animator>(),
E.GetComponent<Animator>() };
}
// 播放动画
public void PlayAnimation()
{
animators[playIndex].Play("YourAnimationStateName"); // 替换 "YourAnimationStateName" 为实际动画状态名
}
// 暂停动画
public void PauseAnimation()
{
animators[playIndex].Pause();
}
// 继续播放动画
public void ResumeAnimation()
{
animators[playIndex].Resume();
}
// 选择下一个对象的动画播放
public void NextAnimation()
{
playIndex = (playIndex + 1) % animators.Length;
}
// 选择上一个对象的动画播放
public void PreviousAnimation()
{
playIndex = (playIndex - 1 + animators.Length) % animators.Length;
}
}
```
在Unity编辑器中,你需要将上述脚本附加到一个GameObject上。然后,将A、B、C、D和E这五个对象分别拖拽到脚本的对应字段中(或者使用代码动态获取)。你需要在Animator组件上设置正确的动画状态名。
同时,你需要创建UI按钮,并将它们与脚本中的方法进行关联。可以通过Unity编辑器的Inspector窗口,使用“Add Component”按钮添加按钮事件触发器,并设置好对应的方法。
确保你的动画状态是在Animator控制器中设置为可控制的,否则`Play`、`Pause`和`Resume`方法将不起作用。"Pause"和"Resume"方法在Animator组件中默认是不可用的,通常需要使用AnimatorControllerParameterType.Bool类型的参数来控制动画的播放和暂停。
阅读全文
相关推荐
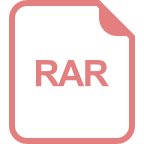
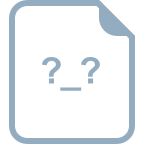















