如何把字符void AddToBuff(char X){//mov x to charbuff f = 0 ; CharBuff[n] = X ;//n is position if (n == 8){//if full uart_disable(); while(1){ if(gpio_get(P_B1) == 0){ uart_enable(); n = 0; uart_tx(' '); f = 1; break; } } } m = n; n++; } void ReadChar(char c){ if (c >= 'A' && c <= 'Z'){ X = c; } },传入这个队列int queue_is_full(Queue *queue) { return ((queue->tail + 1) % queue->size) == (queue->head % queue->size); } int queue_is_empty(Queue *queue) { return queue->tail == queue->head; }
时间: 2024-03-10 18:45:42 浏览: 18
可以将字符添加到队列中,然后通过队列操作将其传递给另一个函数。具体实现如下:
1. 在 AddToBuff 函数中,将字符 X 入队,即调用 queue_enqueue 函数:
```
void AddToBuff(char X){
if(!queue_is_full(&char_queue)) {
queue_enqueue(&char_queue, X);
}
}
```
2. 在 ReadChar 函数中,从队列中读取字符,即调用 queue_dequeue 函数:
```
void ReadChar() {
uint8_t item;
if(!queue_is_empty(&char_queue)) {
queue_dequeue(&char_queue, &item);
if(item >= 'A' && item <= 'Z') {
X = item;
}
}
}
```
需要注意的是,为了让 AddToBuff 和 ReadChar 函数可以访问同一个队列,需要将队列定义为全局变量,并在程序初始化时进行初始化:
```
Queue char_queue;
int main() {
queue_init(&char_queue);
...
return 0;
}
```
相关问题
如何为这个charbuff定义head和tail.void AddToBuff(char X){//mov x to charbuff f = 0 ; CharBuff[n] = X ;//n is position if (n == 8){//if full uart_disable(); while(1){ if(gpio_get(P_B1) == 0){ uart_enable(); n = 0; uart_tx(' '); f = 1; break; } } } m = n; n++; } void ReadChar(char c){ if (c >= 'A' && c <= 'Z'){ X = c; } } void ReadFromBuff(int m){//read buff to m R = CharBuff[m]; }
要为CharBuff定义head(头)和tail(尾),可以在AddToBuff函数中添加两个变量来跟踪头和尾的位置。
例如,可以添加两个名为head和tail的变量来分别表示CharBuff数组中第一个有效字符的位置和下一个要添加字符的位置。在AddToBuff函数中,如果CharBuff数组已经存满了8个字符,则可以将head的值加1,表示第一个字符已经被移除。然后,可以将新的字符存储到CharBuff数组的尾部,并将tail的值设置为新的尾部位置。
下面是修改后的代码:
```c
int head = 0;
int tail = 0;
void AddToBuff(char X) {
if (tail == head && f == 1) { // if full
uart_disable();
while (1) {
if (gpio_get(P_B1) == 0) {
uart_enable();
head = 0;
tail = 0;
uart_tx(' ');
f = 0;
break;
}
}
}
CharBuff[tail] = X;
tail = (tail + 1) % 8;
if (tail == head) {
f = 1;
}
}
void ReadFromBuff(int m) {
int index = (head + m) % 8;
R = CharBuff[index];
}
```
这里使用了%运算符来实现循环队列。在AddToBuff函数中,如果CharBuff数组已满,则将f设置为1,表示队列已满。在ReadFromBuff函数中,使用head和m计算出实际的索引位置,以从CharBuff数组中读取数据。
如何为这个charbuff定义void AddToBuff(char X){//mov x to charbuff f = 0 ; CharBuff[n] = X ;//n is position if (n == 8){//if full uart_disable(); while(1){ if(gpio_get(P_B1) == 0){ uart_enable(); n = 0; uart_tx(' '); f = 1; break; } } } m = n; n++; } void ReadChar(char c){ if (c >= 'A' && c <= 'Z'){ X = c; } } void ReadFromBuff(int m){//read buff to m R = CharBuff[m]; }
这段代码定义了两个函数:AddToBuff和ReadFromBuff,还有一个ReadChar函数用于读取字符并将大写字母存储到变量X中。
AddToBuff函数的作用是将字符X存储到CharBuff数组中的第n个位置,然后将n加1。如果CharBuff数组已经存满了8个字符,那么就禁用UART通信并等待用户按下P_B1引脚。当用户按下P_B1引脚时,启用UART通信,将n重置为0,发送一个空格字符并将f设置为1。最后,将m设置为n。
ReadFromBuff函数的作用是从CharBuff数组中读取第m个位置的字符,并将其存储到R变量中。
相关推荐
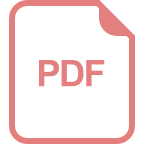
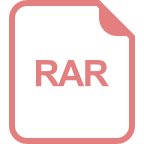














