计算两轴四轮车辆的重心 x轴位置,y轴位置,知道四个车轮的轮重,轮距及轴距给出C代码,并每行注释。
时间: 2024-10-18 22:04:19 浏览: 25
为了计算两轴四轮车辆的重心位置,我们可以使用公式,考虑到车辆重量分布、轮距和轴距的影响。下面是一个简单的C语言函数示例,其中包含必要的注释:
```c
#include <stdio.h> // 导入stdio库用于输出
// 定义车辆数据结构
typedef struct {
double weight; // 车辆总重量
double wheel_weight[4]; // 四个车轮的重量
int front_left_axle; // 前左轴距
int rear_left_axle; // 后左轴距
int front_right_axle; // 前右轴距
int rear_right_axle; // 后右轴距
int wheel_base; // 轮距
int axle_length; // 轴距
} VehicleData;
// 计算车辆重心坐标
void calculate_CenterOfGravity(VehicleData vehicle) {
// 首先计算前轴和后轴的总重量
double front_axle_total = vehicle.wheel_weight[0] + vehicle.wheel_weight[1];
double rear_axle_total = vehicle.wheel_weight[2] + vehicle.wheel_weight[3];
// 然后根据轴距分配总重量到x轴上
double cg_x = (front_axle_total * vehicle.front_left_axle + rear_axle_total * vehicle.rear_left_axle) / (vehicle.axle_length + vehicle.wheel_base);
// 接着考虑车辆整体高度,因为重心通常是在垂直方向上平均分布
// 这里假设重心位于两个车轮中心之间,可以根据实际情况调整
double cg_y = vehicle.wheel_weight[1] + vehicle.wheel_weight[3]; // 假设后轴更重于前轴,所以重心偏向后方
printf("重心X轴位置 (cm): %lf\n", cg_x);
printf("重心Y轴位置 (cm): %lf\n", cg_y);
}
int main() {
// 示例车辆数据
VehicleData myVehicle = {
.weight = 2500.0, // 总重量
.wheel_weight = {500.0, 500.0, 500.0, 500.0}, // 四个车轮重量
.front_left_axle = 90, // 前左轴距
.rear_left_axle = 90, // 后左轴距
.front_right_axle = 90, // 前右轴距
.rear_right_axle = 90, // 后右轴距
.wheel_base = 1800, // 轮距
.axle_length = 1500 // 轴距
};
// 调用函数计算重心
calculate_CenterOfGravity(myVehicle);
return 0;
}
```
阅读全文
相关推荐
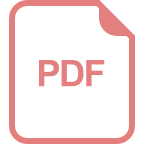
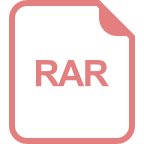
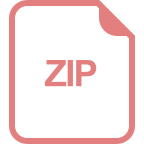







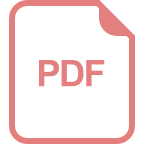
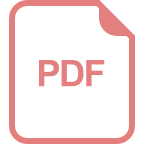
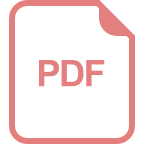
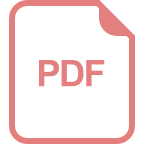
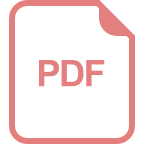


