用Python写一个圆周率派的近似值,其中n=1,2,3......计算n=1000
时间: 2023-03-27 21:02:41 浏览: 190
可以使用蒙特卡罗方法来近似计算圆周率派。具体实现如下:
```python
import random
def approximate_pi(n):
count =
for i in range(n):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
if x**2 + y**2 <= 1:
count += 1
return 4 * count / n
print(approximate_pi(100))
```
其中,我们在[-1, 1]范围内随机生成x和y坐标,如果它们的距离原点的距离小于等于1,则认为它们在圆内,否则在圆外。统计在圆内的点的数量,最后用这个数量除以总的点数,再乘以4,就可以得到圆周率派的近似值。
相关问题
python输入n计算圆周率的近似值
可以使用蒙特卡洛方法计算圆周率的近似值。下面是使用 Python 实现的代码:
```python
import random
def estimate_pi(n):
num_inside = 0
for i in range(n):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
if x**2 + y**2 < 1:
num_inside += 1
return 4 * num_inside / n
print(estimate_pi(1000000))
```
该方法的原理是,随机生成一些坐标点,计算它们距离中心点的距离,如果小于半径 1,则这个点在圆内,否则在圆外。然后统计在圆内的点的数量,除以总点数,再乘以 4 就是圆周率的近似值。
需要注意的是,这个近似值的精度随着计算的点数增加而增加,但是随机生成的点的数量太少可能会导致结果不准确。
如何使用Python编程语言,基于公式 π/4 ≈ 1 - 1/3 + 1/5 - 1/7 + ... + (-1)^(n-1) / n (其中n=1000),来计算圆周率π的一个近似值?
在Python中,你可以编写一个简单的程序来利用该无穷级数公式计算圆周率π的近似值。以下是步骤:
1. 首先,创建一个函数,名为`pi_approximation`,它接受一个整数`n`作为参数,表示级数中的项数。
```python
def pi_approximation(n):
```
2. 使用循环结构,从1开始迭代到n,每次检查当前项是否为奇数,然后应用给定的正负符号。
```python
result = 0
term = 1
sign = 1
for i in range(1, n+1):
result += sign * (1 / i)
sign *= -1 # 每次改变符号
```
3. 将结果乘以4,因为级数公式里的π/4。
```python
result *= 4
```
4. 返回结果,这就是π的近似值。
```python
return result
```
完整代码如下:
```python
def pi_approximation(n):
result = 0
term = 1
sign = 1
for i in range(1, n+1):
result += sign * (1 / i)
sign *= -1 # 每次改变符号
result *= 4
return result
# 计算π的近似值
approx_pi = pi_approximation(1000)
approx_pi
```
阅读全文
相关推荐
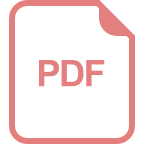













