计算n!算法如下,请改正程序中的错误,使它能得出正确的结果。 #include <stdio.h> long fact(int n); main() { int n, result = 0; printf("Input n:"); scanf("%d", &n); result = fact(n); printf("%d != %d", n, result); } long fact(int n) { int result; if (n < 0) printf("n<0,data error!\n"); else { result = n * fact(n - 1); return result; } }
时间: 2024-03-26 14:36:02 浏览: 101
这段程序存在两个问题:
1. 在fact函数中,缺少结束递归的条件,导致递归调用无法结束,程序会出现错误或死循环。
2. 在主函数中,result的初始值应该是1而不是0,否则最终结果会偏小。
以下是修改后的程序:
```c
#include <stdio.h>
long fact(int n);
int main() {
int n, result = 1;
printf("Input n:");
scanf("%d", &n);
result = fact(n);
printf("%d != %ld", n, result);
return 0;
}
long fact(int n) {
long result;
if (n < 0) {
printf("n<0,data error!\n");
return -1; // 返回一个错误代码
} else if (n == 0) {
return 1; // 结束递归
} else {
result = n * fact(n - 1);
return result;
}
}
```
修改后的程序中,在fact函数中增加了结束递归的条件(当n等于0时),同时在主函数中将result的初始值改为1。这样程序就能正确计算n的阶乘了,并且在输入负数时会返回一个错误代码-1。
相关问题
计算n!算法如下,请改正程序中的错误,使它能得出正确的结果。 #include <stdio.h> long fact(int n); main() { int n, result = 0; printf("Input n:"); scanf("%d", &n); result = fact(n); printf("%d != %d", n, result); } long fact(int n) { int result; if (
程序截断了,我不知道你的具体问题是什么。但是根据你提供的代码,可以看出以下错误:
1. 在主函数中,将result初始化为0是无意义的,因为阶乘的结果不可能为0。
2. 在计算阶乘的函数fact中,没有给result赋初值,导致计算结果不确定。
3. 在计算阶乘的函数fact中,没有设置递归结束的条件,会导致无限递归。
下面是改正后的程序:
```
#include <stdio.h>
long fact(int n);
int main() {
int n;
printf("Input n:");
scanf("%d", &n);
printf("%d! = %ld", n, fact(n));
return 0;
}
long fact(int n) {
if (n == 1 || n == 0) {
return 1;
} else {
return n * fact(n-1);
}
}
```
改正后的程序中,将result的初值设为1,因为阶乘的结果一般不为0,并且在计算阶乘的函数fact中加入了递归结束条件,保证了程序的正确性。
2.编程从键盘输入n值(3≤n≤10),然后计算并输出二i !=1 !+2! +3 ! +..+!. .[问题分析] 计算1 ! +2 ! +3 ! ...+n!相对于计算1+1x2+1x2*3+...+1x2x3...xn. 问题就是求阶乘和的值,使用双重循环即可实现。 [算法设计] (1)外循环控制变量为i,其变化范围从1到n,依次计算从1到n的各个阶乘值的累 加求和; (2)内循环控制变量为j.其变化范围从1到i,依次计算从1到i的累积,即阶乘n!.[代码实现] 请根据算法写出完整的程序,并编译、 运行、检查结果的正确性。
以下是求阶乘和的解法:
```
#include <stdio.h>
int main() {
int n, i, j;
long long sum = 0, fact = 1;
printf("请输入n的值(3<=n<=10):");
scanf("%d", &n);
if (n < 3 || n > 10) {
printf("n的值必须在3到10之间\n");
return 1;
}
for (i = 1; i <= n; i++) {
fact = 1;
for (j = 1; j <= i; j++) {
fact *= j;
}
sum += fact;
}
printf("从1到%d的阶乘和为%lld\n", n, sum);
return 0;
}
```
这个程序实现了上述的算法设计,首先从键盘读取n的值,然后进行输入验证以确保n的值在3到10之间,接着按照算法设计的方案计算阶乘和,最后输出结果。注意,我们使用了long long类型来存储阶乘和,因为这个值可能会非常大。
阅读全文
相关推荐
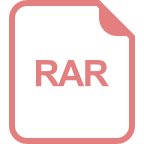
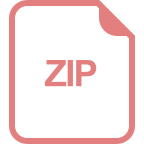
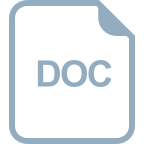
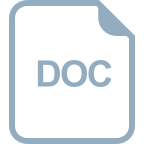
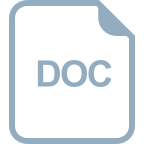









