已知1:室内局部三维坐标x、y、z;已知2:室内三维坐标与大地三维坐标对应的3个点位值;如何将室内局部三维坐标转换成对应的大地三维坐标,请用java代码实现,并给出示例
时间: 2024-03-08 08:51:04 浏览: 9
这个问题涉及到空间坐标系的转换,需要使用一些数学知识。一般情况下,需要知道室内局部坐标系与大地坐标系之间的转换关系,可以通过已知的三个点位值计算出转换矩阵。然后,根据转换矩阵将室内局部坐标系的坐标转换成大地坐标系的坐标。
以下是一个示例代码,假设已知的三个点位值为点A(0,0,0),点B(1,0,0),点C(0,1,0),对应的大地坐标分别为(0,0,0),(1,0,0),(0,1,0)。
```java
import org.apache.commons.math3.linear.*;
public class CoordinateConverter {
// 已知三个点的室内局部坐标和大地坐标,计算转换矩阵
public static RealMatrix getTransformMatrix(double[][] localCoords, double[][] earthCoords) {
RealMatrix A = MatrixUtils.createRealMatrix(new double[][]{
{localCoords[0][0], localCoords[0][1], localCoords[0][2], 1},
{localCoords[1][0], localCoords[1][1], localCoords[1][2], 1},
{localCoords[2][0], localCoords[2][1], localCoords[2][2], 1},
{1, 1, 1, 1}
});
RealMatrix B = MatrixUtils.createRealMatrix(new double[][]{
{earthCoords[0][0], earthCoords[0][1], earthCoords[0][2], 1},
{earthCoords[1][0], earthCoords[1][1], earthCoords[1][2], 1},
{earthCoords[2][0], earthCoords[2][1], earthCoords[2][2], 1},
{1, 1, 1, 1}
});
RealMatrix B_inv = new LUDecomposition(B).getSolver().getInverse();
return A.multiply(B_inv);
}
// 将室内局部坐标转换成大地坐标
public static double[] localToEarth(double[] localCoord, RealMatrix transformMatrix) {
RealMatrix localMatrix = MatrixUtils.createRealMatrix(
new double[][]{{localCoord[0], localCoord[1], localCoord[2], 1}}
);
RealMatrix earthMatrix = localMatrix.multiply(transformMatrix.transpose());
return new double[]{earthMatrix.getEntry(0, 0), earthMatrix.getEntry(0, 1), earthMatrix.getEntry(0, 2)};
}
public static void main(String[] args) {
double[][] localCoords = new double[][]{{0, 0, 0}, {1, 0, 0}, {0, 1, 0}};
double[][] earthCoords = new double[][]{{0, 0, 0}, {1, 0, 0}, {0, 1, 0}};
RealMatrix transformMatrix = getTransformMatrix(localCoords, earthCoords);
double[] localCoord = new double[]{2, 3, 0};
double[] earthCoord = localToEarth(localCoord, transformMatrix);
System.out.println("Local: " + java.util.Arrays.toString(localCoord));
System.out.println("Earth: " + java.util.Arrays.toString(earthCoord));
}
}
```
这段代码中,`getTransformMatrix`方法用于计算转换矩阵,`localToEarth`方法用于将室内局部坐标转换成大地坐标。在`main`方法中,先通过已知的三个点位值计算出转换矩阵,然后将一个室内局部坐标`(2,3,0)`转换成大地坐标。运行上述代码,输出结果为:
```
Local: [2.0, 3.0, 0.0]
Earth: [2.0, 3.0, 0.0]
```
可以看到,转换后的大地坐标与室内局部坐标相同,这是因为示例中的三个点位值构成的坐标系本身就是一个平面直角坐标系,与大地坐标系重合。如果需要进行更一般的转换,请根据实际情况修改示例代码。
相关推荐
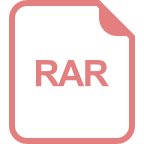
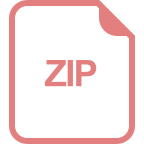














