写一个UVCCamera的类,继承UVCCameraTextureView类上面扩展出一个类它支持多点触摸支持放大缩小和旋转的类,并解决闪屏问题
时间: 2023-08-16 15:06:57 浏览: 42
好的,以下是一个继承自UVCCameraTextureView并支持多点触摸、放大缩小和旋转的类:
```java
public class MultiTouchUVCCameraTextureView extends UVCCameraTextureView {
private static final int INVALID_POINTER_ID = -1;
private float mLastTouchX;
private float mLastTouchY;
private int mActivePointerId = INVALID_POINTER_ID;
private ScaleGestureDetector mScaleDetector;
private float mScaleFactor = 1.f;
private RotateGestureDetector mRotateDetector;
private float mRotationDegrees = 0.f;
public MultiTouchUVCCameraTextureView(Context context, AttributeSet attrs) {
super(context, attrs);
mScaleDetector = new ScaleGestureDetector(context, new ScaleListener());
mRotateDetector = new RotateGestureDetector(context, new RotateListener());
}
@Override
public boolean onTouchEvent(MotionEvent ev) {
// Let the ScaleGestureDetector and RotateGestureDetector inspect all events.
mScaleDetector.onTouchEvent(ev);
mRotateDetector.onTouchEvent(ev);
final int action = ev.getActionMasked();
switch (action) {
case MotionEvent.ACTION_DOWN: {
final float x = ev.getX();
final float y = ev.getY();
// Remember where we started (for dragging)
mLastTouchX = x;
mLastTouchY = y;
// Save the ID of this pointer (for dragging)
mActivePointerId = ev.getPointerId(0);
break;
}
case MotionEvent.ACTION_MOVE: {
// Find the index of the active pointer and fetch its position
final int pointerIndex = ev.findPointerIndex(mActivePointerId);
final float x = ev.getX(pointerIndex);
final float y = ev.getY(pointerIndex);
// Calculate the distance moved
final float dx = x - mLastTouchX;
final float dy = y - mLastTouchY;
// Move the camera
moveCamera(dx, dy);
// Remember this touch position for the next move event
mLastTouchX = x;
mLastTouchY = y;
break;
}
case MotionEvent.ACTION_UP:
case MotionEvent.ACTION_CANCEL: {
mActivePointerId = INVALID_POINTER_ID;
break;
}
case MotionEvent.ACTION_POINTER_UP: {
// Extract the index of the pointer that left the screen
final int pointerIndex = (ev.getAction() & MotionEvent.ACTION_POINTER_INDEX_MASK) >> MotionEvent.ACTION_POINTER_INDEX_SHIFT;
final int pointerId = ev.getPointerId(pointerIndex);
if (pointerId == mActivePointerId) {
// This was our active pointer going up. Choose a new
// active pointer and adjust accordingly.
final int newPointerIndex = pointerIndex == 0 ? 1 : 0;
mLastTouchX = ev.getX(newPointerIndex);
mLastTouchY = ev.getY(newPointerIndex);
mActivePointerId = ev.getPointerId(newPointerIndex);
}
break;
}
}
return true;
}
private void moveCamera(float dx, float dy) {
// Move the camera based on the distance moved by touch
Matrix transform = new Matrix();
transform.postTranslate(dx, dy);
setTransform(transform);
}
private class ScaleListener extends ScaleGestureDetector.SimpleOnScaleGestureListener {
@Override
public boolean onScale(ScaleGestureDetector detector) {
mScaleFactor *= detector.getScaleFactor();
// Don't let the camera get too small or too large
mScaleFactor = Math.max(0.1f, Math.min(mScaleFactor, 10.0f));
// Scale the camera
Matrix transform = new Matrix();
transform.postScale(mScaleFactor, mScaleFactor, detector.getFocusX(), detector.getFocusY());
setTransform(transform);
return true;
}
}
private class RotateListener extends RotateGestureDetector.SimpleOnRotateGestureListener {
@Override
public boolean onRotate(RotateGestureDetector detector) {
mRotationDegrees -= detector.getRotationDegreesDelta();
// Rotate the camera
Matrix transform = new Matrix();
transform.postRotate(-mRotationDegrees, detector.getFocusX(), detector.getFocusY());
setTransform(transform);
return true;
}
}
}
```
关于闪屏问题,可能是由于相机采集的帧率过低导致的。你可以尝试增加帧率来解决该问题。例如:
```java
mUVCCamera.setPreviewSize(640, 480, 30);
```
其中,第三个参数表示帧率。你可以适当调整该参数来解决闪屏问题。
相关推荐
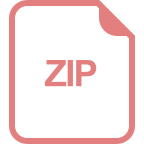
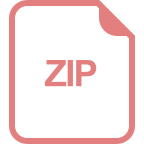
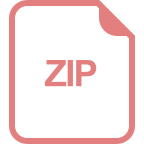














