import heapq import copy # 定义状态类 class State: def __init__(self, board, moves=0, parent=None, last_move=None): self.board = board self.moves = moves self.parent = parent self.last_move = last_move def __lt__(self, other): return self.moves < other.moves def __eq__(self, other): return self.board == other.board # 定义转移函数 def move(state, direction): new_board = copy.deepcopy(state.board) for i in range(len(new_board)): if 0 in new_board[i]: j = new_board[i].index(0) break if direction == "up": if i == 0: return None else: new_board[i][j], new_board[i-1][j] = new_board[i-1][j], new_board[i][j] elif direction == "down": if i == len(new_board)-1: return None else: new_board[i][j], new_board[i+1][j] = new_board[i+1][j], new_board[i][j] elif direction == "left": if j == 0: return None else: new_board[i][j], new_board[i][j-1] = new_board[i][j-1], new_board[i][j] elif direction == "right": if j == len(new_board)-1: return None else: new_board[i][j], new_board[i][j+1] = new_board[i][j+1], new_board[i][j] return State(new_board, state.moves+1, state, direction) # 定义A*算法 def astar(start, goal): heap = [] closed = set() heapq.heappush(heap, start) while heap: state = heapq.heappop(heap) if state.board == goal: path = [] while state.parent: path.append(state) state = state.parent path.append(state) return path[::-1] closed.add(state) for direction in ["up", "down", "left", "right"]: child = move(state, direction) if child is None: continue if child in closed: continue if child not in heap: heapq.heappush(heap, child) else: for i, (p, c) in enumerate(heap): if c == child and p.moves > child.moves: heap[i] = (child, child) heapq.heapify(heap) # 测试 start_board = [[1, 2, 3], [4, 5, 6], [7, 8, 0]] goal_board = [[2, 3, 6], [1, 5, 8], [4, 7, 0]] start_state = State(start_board) goal_state = State(goal_board) path = astar(start_state, goal_board) for state in path: print(state.board) 这段代码运行后报错,因为State是不可hash的,那么如何修改才能使得功能一样且能够运行
时间: 2023-06-01 08:01:38 浏览: 133
import heapq 和 import copy 是 Python 中的两个模块。
import heapq 用于实现堆数据结构。堆是一种特殊的树形数据结构,它满足堆的性质:对于每个节点,其父节点的值都比其子节点的值要小(或大,具体取决于堆的类型)。堆可以用来实现优先队列,即每次弹出的元素都是优先级最高的。
import copy 用于实现复制操作。在 Python 中,变量之间的赋值实际上是将一个对象的引用赋给另一个变量,而不是将对象本身复制一份。因此,如果要复制一个对象并在原对象的基础上进行修改,就需要使用 copy 模块中的函数进行复制操作。copy 模块中提供了浅复制和深复制两种方式,具体的区别可以参见官方文档。
相关问题
import heapq import copy # 定义状态类 class State: def __init__(self, board, moves=0, parent=None, last_move=None): self.board = board self.moves = moves self.parent = parent self.last_move = last_move def __lt__(self, other): return self.moves < other.moves def __eq__(self, other): return self.board == other.board # 定义转移函数 def move(state, direction): new_board = copy.deepcopy(state.board) for i in range(len(new_board)): if 0 in new_board[i]: j = new_board[i].index(0) break if direction == "up": if i == 0: return None else: new_board[i][j], new_board[i-1][j] = new_board[i-1][j], new_board[i][j] elif direction == "down": if i == len(new_board)-1: return None else: new_board[i][j], new_board[i+1][j] = new_board[i+1][j], new_board[i][j] elif direction == "left": if j == 0: return None else: new_board[i][j], new_board[i][j-1] = new_board[i][j-1], new_board[i][j] elif direction == "right": if j == len(new_board)-1: return None else: new_board[i][j], new_board[i][j+1] = new_board[i][j+1], new_board[i][j] return State(new_board, state.moves+1, state, direction) # 定义A*算法 def astar(start, goal): heap = [] closed = set() heapq.heappush(heap, start) while heap: state = heapq.heappop(heap) if state.board == goal: path = [] while state.parent: path.append(state) state = state.parent path.append(state) return path[::-1] closed.add(state) for direction in ["up", "down", "left", "right"]: child = move(state, direction) if child is None: continue if child in closed: continue if child not in heap: heapq.heappush(heap, child) else: for i, (p, c) in enumerate(heap): if c == child and p.moves > child.moves: heap[i] = (child, child) heapq.heapify(heap) # 测试 start_board = [[1, 2, 3], [4, 5, 6], [7, 8, 0]] goal_board = [[2, 3, 6], [1, 5, 8], [4, 7, 0]] start_state = State(start_board) goal_state = State(goal_board) path = astar(start_state, goal_board) for state in path: print(state.board)
import heapq 是 Python 中用于实现堆数据结构的模块,可以用于排序和优先队列等场景。而 import copy 则是 Python 中用于复制对象的模块,可以使用 deepcopy 和 shallowcopy 等方法来实现深拷贝和浅拷贝。
class FixedSizeHeap: def __init__(self, max_size): self.max_size = max_size self.heap = [] def push(self, item): if len(self.heap) < self.max_size: heapq.heappush(self.heap, item) else: min_item = heapq.heappop(self.heap) if item > min_item: heapq.heappush(self.heap, item) else: heapq.heappush(self.heap, min_item) def pop(self): return heapq.heappop(self.heap) def __len__(self): return len(self.heap)对于这样的类怎么打印类中的元素
要打印类中的元素,可以通过实现`__str__`方法来实现。`__str__`方法应该返回一个字符串,该字符串包含对象的信息。例如,以下代码演示了如何在类中实现`__str__`方法以打印堆的元素:
```
import heapq
class FixedSizeHeap:
def __init__(self, max_size):
self.max_size = max_size
self.heap = []
def push(self, item):
if len(self.heap) < self.max_size:
heapq.heappush(self.heap, item)
else:
min_item = heapq.heappop(self.heap)
if item > min_item:
heapq.heappush(self.heap, item)
else:
heapq.heappush(self.heap, min_item)
def pop(self):
return heapq.heappop(self.heap)
def __len__(self):
return len(self.heap)
def __str__(self):
return str(self.heap)
new_heap = FixedSizeHeap(5)
new_heap.push(1)
new_heap.push(2)
new_heap.push(3)
new_heap.push(4)
new_heap.push(5)
print(new_heap) # 输出 [1, 2, 3, 4, 5]
```
在这个例子中,我们定义了`__str__`方法来返回堆的元素。我们将`self.heap`转换为字符串并返回。现在,当我们打印`new_heap`时,它将打印堆中的元素。
阅读全文
相关推荐
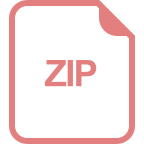
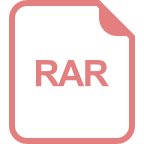
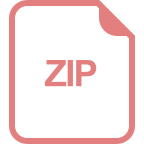














