需要写一段俄罗斯方块的程序
时间: 2023-09-18 08:13:00 浏览: 49
好的,下面是一个简单的俄罗斯方块程序,使用 Python 语言编写:
```python
import pygame
import random
# 定义方块的大小和游戏区域的大小
BLOCK_SIZE = 30
SCREEN_WIDTH = 10 * BLOCK_SIZE
SCREEN_HEIGHT = 20 * BLOCK_SIZE
# 定义方块的形状,用二维数组表示
SHAPE_I = [[1, 1, 1, 1]]
SHAPE_L = [[1, 0, 0], [1, 1, 1]]
SHAPE_J = [[0, 0, 1], [1, 1, 1]]
SHAPE_O = [[1, 1], [1, 1]]
SHAPE_S = [[0, 1, 1], [1, 1, 0]]
SHAPE_T = [[0, 1, 0], [1, 1, 1]]
SHAPE_Z = [[1, 1, 0], [0, 1, 1]]
SHAPES = [SHAPE_I, SHAPE_L, SHAPE_J, SHAPE_O, SHAPE_S, SHAPE_T, SHAPE_Z]
COLORS = [(0, 255, 255), (255, 0, 255), (255, 255, 0), (0, 0, 255), (0, 255, 0), (255, 140, 0), (255, 0, 0)]
# 初始化 pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置窗口标题
pygame.display.set_caption('俄罗斯方块')
# 定义方块类
class Block:
def __init__(self, shape, color, x, y):
self.shape = shape
self.color = color
self.x = x
self.y = y
# 绘制方块
def draw(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
pygame.draw.rect(screen, self.color, (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 左移
def move_left(self):
self.x -= BLOCK_SIZE
# 右移
def move_right(self):
self.x += BLOCK_SIZE
# 下落
def move_down(self):
self.y += BLOCK_SIZE
# 定义游戏区域类
class GameArea:
def __init__(self):
self.blocks = []
self.current_block = None
self.next_block = None
self.score = 0
# 随机生成一个方块
def generate_block(self):
shape = random.choice(SHAPES)
color = random.choice(COLORS)
x = SCREEN_WIDTH // 2 - 2 * BLOCK_SIZE
y = -2 * BLOCK_SIZE
self.current_block = Block(shape, color, x, y)
shape = random.choice(SHAPES)
color = random.choice(COLORS)
x = SCREEN_WIDTH + 4 * BLOCK_SIZE
y = SCREEN_HEIGHT // 2 - 2 * BLOCK_SIZE
self.next_block = Block(shape, color, x, y)
# 向下移动当前方块
def move_current_block_down(self):
self.current_block.move_down()
if self.check_collision():
self.current_block.move_up()
self.add_block_to_blocks()
self.generate_block()
self.clear_lines()
# 检测当前方块是否与已有方块重叠
def check_collision(self):
for block in self.blocks:
for i in range(len(self.current_block.shape)):
for j in range(len(self.current_block.shape[i])):
if self.current_block.shape[i][j] == 1:
x = self.current_block.x + j * BLOCK_SIZE
y = self.current_block.y + i * BLOCK_SIZE
if x == block.x and y == block.y:
return True
return self.current_block.y + len(self.current_block.shape) * BLOCK_SIZE > SCREEN_HEIGHT
# 将当前方块加入已有方块列表中
def add_block_to_blocks(self):
for i in range(len(self.current_block.shape)):
for j in range(len(self.current_block.shape[i])):
if self.current_block.shape[i][j] == 1:
x = self.current_block.x + j * BLOCK_SIZE
y = self.current_block.y + i * BLOCK_SIZE
block = Block(self.current_block.shape, self.current_block.color, x, y)
self.blocks.append(block)
# 消除满行
def clear_lines(self):
y_values = [block.y for block in self.blocks]
y_values = list(set(y_values))
y_values.sort(reverse=True)
for y in y_values:
line_blocks = [block for block in self.blocks if block.y == y]
if len(line_blocks) == SCREEN_WIDTH // BLOCK_SIZE:
self.score += 10
for block in line_blocks:
self.blocks.remove(block)
for block in self.blocks:
if block.y < y:
block.move_down()
# 绘制游戏区域
def draw(self):
screen.fill((0, 0, 0))
pygame.draw.rect(screen, (255, 255, 255), (0, 0, SCREEN_WIDTH, SCREEN_HEIGHT), 1)
for block in self.blocks:
block.draw()
self.current_block.draw()
self.next_block.draw()
font = pygame.font.Font(None, 30)
text = font.render('Score: {}'.format(self.score), True, (255, 255, 255))
screen.blit(text, (10, 10))
# 暂停游戏
def pause(self):
font = pygame.font.Font(None, 50)
text = font.render('PAUSE', True, (255, 255, 255))
screen.blit(text, (SCREEN_WIDTH // 2 - 70, SCREEN_HEIGHT // 2 - 25))
pygame.display.flip()
while True:
for event in pygame.event.get():
if event.type == pygame.KEYDOWN and event.key == pygame.K_SPACE:
return
# 游戏结束
def game_over(self):
font = pygame.font.Font(None, 50)
text = font.render('GAME OVER', True, (255, 255, 255))
screen.blit(text, (SCREEN_WIDTH // 2 - 120, SCREEN_HEIGHT // 2 - 25))
pygame.display.flip()
pygame.time.delay(2000)
pygame.quit()
quit()
# 创建游戏区域实例
game_area = GameArea()
# 随机生成一个方块
game_area.generate_block()
# 游戏循环
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_p:
game_area.pause()
elif event.key == pygame.K_LEFT:
game_area.current_block.move_left()
if game_area.check_collision():
game_area.current_block.move_right()
elif event.key == pygame.K_RIGHT:
game_area.current_block.move_right()
if game_area.check_collision():
game_area.current_block.move_left()
elif event.key == pygame.K_DOWN:
game_area.move_current_block_down()
elif event.key == pygame.K_SPACE:
while not game_area.check_collision():
game_area.move_current_block_down()
# 向下移动当前方块
game_area.move_current_block_down()
# 绘制游戏区域
game_area.draw()
# 显示窗口
pygame.display.flip()
# 判断游戏是否结束
for block in game_area.blocks:
if block.y <= 0:
game_area.game_over()
# 控制帧率
clock.tick(5)
```
在运行这个程序之前,需要先安装 Pygame 库。可以使用以下命令来安装:
```
pip install pygame
```
运行程序之后,按左右方向键可以控制方块的移动,按下方向键可以加速方块的下落,按下空格键可以让方块直接落到底部。按下 P 键可以暂停游戏。当方块堆到游戏区域的顶部时,游戏结束。
相关推荐
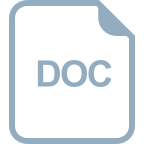
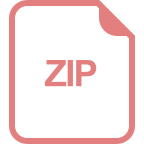
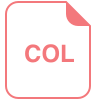
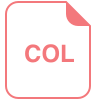
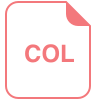
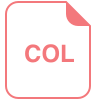
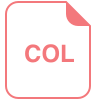









