用clojure写一个德州扑克游戏
时间: 2023-12-18 17:05:18 浏览: 36
好的,以下是一个简单的Clojure实现的德州扑克游戏:
```clojure
(ns texas-holdem.core
(:gen-class))
(def suits [:hearts :diamonds :clubs :spades])
(def ranks [:two :three :four :five :six :seven :eight :nine :ten :jack :queen :king :ace])
(defn shuffle-deck []
(shuffle (for [suit suits
rank ranks]
{:suit suit
:rank rank})))
(defn deal [players deck]
(loop [p players
d deck
hands []]
(if (empty? p)
hands
(let [hand (take 2 d)]
(recur (rest p) (drop 2 d) (conj hands (vec hand)))))))
(defn flop [deck]
(let [cards (take 3 deck)
rest (drop 3 deck)]
[cards rest]))
(defn turn [deck]
(let [card (first deck)
rest (rest deck)]
[card rest]))
(defn river [deck]
(let [card (first deck)
rest (rest deck)]
[card rest]))
(defn best-hand [cards]
(let [combinations (sequence 5 (subseq cards 0))
hands (map #(sort-by :rank %) (sequence 10 (combinations combinations)))
flushes (filter #(= (count (distinct (map :suit %))) 1) hands)
straights (filter #(= (count (distinct (map :rank %))) 5) hands)
straight-flushes (filter #(and (= (count (distinct (map :suit %))) 1) (= (count (distinct (map :rank %))) 5)) hands)
royal-flushes (filter #(= (count (distinct (map :rank %))) 5) straight-flushes)]
(if (not-empty royal-flushes)
{:hand :royal-flush
:cards (first royal-flushes)}
(if (not-empty straight-flushes)
{:hand :straight-flush
:cards (first straight-flushes)}
(if (not-empty four-of-a-kinds)
{:hand :four-of-a-kind
:cards (first four-of-a-kinds)}
(if (not-empty full-houses)
{:hand :full-house
:cards (first full-houses)}
(if (not-empty flushes)
{:hand :flush
:cards (first flushes)}
(if (not-empty straights)
{:hand :straight
:cards (first straights)}
(if (not-empty three-of-a-kinds)
{:hand :three-of-a-kind
:cards (first three-of-a-kinds)}
(if (not-empty two-pairs)
{:hand :two-pair
:cards (first two-pairs)}
(if (not-empty pairs)
{:hand :pair
:cards (first pairs)}
{:hand :high-card
:cards (first hands)})))))))))))
(defn winner [hands]
(let [best (apply max-key #(best-hand %) hands)]
(filter #(= best (best-hand %)) hands)))
(defn -main []
(let [players (list ["Player 1" :bankroll 100]
["Player 2" :bankroll 100])
deck (shuffle-deck)
hands (deal players deck)
[flop deck] (flop deck)
[turn deck] (turn deck)
[river deck] (river deck)
player-hands (map #(conj % flop turn river) hands)
winners (winner player-hands)]
(println "Winning hand:" (best-hand (first winners)))
(doseq [[hand player] (map vector player-hands players)]
(println player "has" hand))
(doseq [w winners]
(println (first w) "wins!"))))
```
这个程序实现了一个简单的德州扑克游戏,包括洗牌、发牌、翻牌、转牌、河牌、计算最佳手牌和决定获胜者等功能。你可以在此基础上进行扩展,添加更多的游戏规则和功能。
相关推荐
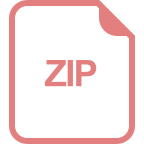
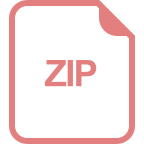
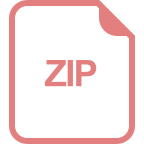









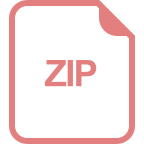
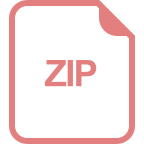
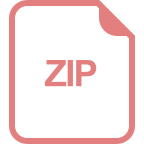
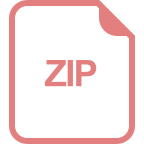
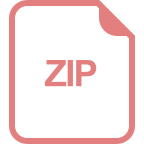