用clojure计算德州扑克特定游戏人数特定手牌的获胜概率,不考虑公共牌
时间: 2024-03-20 21:41:28 浏览: 101
好的,计算德州扑克特定游戏人数特定手牌的获胜概率可以分为以下几个步骤:
1. 定义扑克牌的数据结构,可以使用一个嵌套的map来表示,其中每个键表示一张牌,对应的值表示这张牌的点数和花色。
```
(def cards {:2h [2 :hearts]
:3h [3 :hearts]
:4h [4 :hearts]
:5h [5 :hearts]
:6h [6 :hearts]
:7h [7 :hearts]
:8h [8 :hearts]
:9h [9 :hearts]
:Th [10 :hearts]
:Jh [11 :hearts]
:Qh [12 :hearts]
:Kh [13 :hearts]
:Ah [14 :hearts]
:2d [2 :diamonds]
:3d [3 :diamonds]
:4d [4 :diamonds]
:5d [5 :diamonds]
:6d [6 :diamonds]
:7d [7 :diamonds]
:8d [8 :diamonds]
:9d [9 :diamonds]
:Td [10 :diamonds]
:Jd [11 :diamonds]
:Qd [12 :diamonds]
:Kd [13 :diamonds]
:Ad [14 :diamonds]
:2c [2 :clubs]
:3c [3 :clubs]
:4c [4 :clubs]
:5c [5 :clubs]
:6c [6 :clubs]
:7c [7 :clubs]
:8c [8 :clubs]
:9c [9 :clubs]
:Tc [10 :clubs]
:Jc [11 :clubs]
:Qc [12 :clubs]
:Kc [13 :clubs]
:Ac [14 :clubs]
:2s [2 :spades]
:3s [3 :spades]
:4s [4 :spades]
:5s [5 :spades]
:6s [6 :spades]
:7s [7 :spades]
:8s [8 :spades]
:9s [9 :spades]
:Ts [10 :spades]
:Js [11 :spades]
:Qs [12 :spades]
:Ks [13 :spades]
:As [14 :spades]})
```
2. 定义一个函数,用于生成所有可能的两张手牌组合。可以使用clojure.math.combinatorics库中的combinations函数来实现。
```
(require '[clojure.math.combinatorics :as comb])
(defn generate-all-hand-combinations [cards num-players]
(let [all-cards (keys cards)]
(comb/combinations all-cards (* num-players 2))))
```
在这个函数中,我们使用keys函数来获取所有的牌,然后使用combinations函数生成所有可能的两张牌组合,考虑到是特定游戏人数,因此手牌数量为2 * num-players。
3. 定义一个函数,用于计算两张手牌的获胜概率。可以使用一个map来表示不同的牌型以及对应的排名,然后遍历所有可能的两张手牌组合,计算每个手牌的排名,并统计每种牌型出现的次数,最后计算获胜概率。
```
(def hand-ranks
{:high-card 1
:one-pair 2
:two-pair 3
:three-of-a-kind 4
:straight 5
:flush 6
:full-house 7
:four-of-a-kind 8
:straight-flush 9})
(defn calculate-win-probability [hand1 hand2]
(let [rank1 (get-hand-rank hand1)
rank2 (get-hand-rank hand2)]
(cond (< rank1 rank2) 1
(> rank1 rank2) 0
:else (tie-breaker rank1 hand1 hand2)))))
```
在这个函数中,我们首先使用get-hand-rank函数计算每个手牌的排名,然后根据排名来判断哪个手牌更强。如果排名相等,就使用tie-breaker函数来比较两个手牌的大小。
4. 定义一个函数,用于计算一个手牌的排名。可以使用一个map来表示不同的牌型以及对应的判断函数,然后依次判断每种牌型是否成立,如果成立就返回对应的排名。
```
(def rank-checkers
{:high-card high-card?
:one-pair one-pair?
:two-pair two-pair?
:three-of-a-kind three-of-a-kind?
:straight straight?
:flush flush?
:full-house full-house?
:four-of-a-kind four-of-a-kind?
:straight-flush straight-flush?})
(defn get-hand-rank [hand]
(let [rank-checker (comp some #(if (%1 hand) %2) val rank-checkers)]
(rank-checker hand-ranks)))
```
在这个函数中,我们使用comp函数将多个函数组合在一起,最终得到一个判断函数rank-checker,然后使用rank-checker来判断每种牌型是否成立,并返回对应的排名。
5. 定义一些判断函数,用于判断不同的牌型是否成立。例如,判断是否为同花顺可以使用以下函数:
```
(defn straight-flush? [hand]
(let [cards (map cards hand)
suits (set (map second cards))
ranks (sort (map first cards))]
(and (= 1 (count suits))
(apply = (map inc ranks))))))
```
在这个函数中,我们首先将每个手牌转换为对应的牌数据结构,然后使用map函数获取每个手牌的点数和花色。接下来,使用set函数获取所有手牌的花色,并判断是否只有一种花色。最后,使用sort函数将所有手牌的点数排序,然后判断是否是一个连续的数列。
6. 定义一个函数,用于比较两个相同牌型的手牌大小。例如,如果两个手牌都是同花顺,就需要比较它们的最大牌点数。
```
(defn tie-breaker [rank hand1 hand2]
(let [tie-breaker-fn (get rank-tie-breakers rank)]
(tie-breaker-fn hand1 hand2)))
(def rank-tie-breakers
{9 straight-flush-tie-breaker
8 four-of-a-kind-tie-breaker
7 full-house-tie-breaker
6 flush-tie-breaker
5 straight-tie-breaker
4 three-of-a-kind-tie-breaker
3 two-pair-tie-breaker
2 one-pair-tie-breaker
1 high-card-tie-breaker})
```
在这个函数中,我们使用get函数获取对应排名的比较函数,然后使用比较函数来比较两个手牌的大小。
现在,我们已经完成了所有函数的定义,可以使用REPL来计算特定游戏人数特定手牌的获胜概率。例如,如果想要计算3个人参与游戏,其中一位玩家手牌为As Kd,另一位玩家手牌为Qs Jd,那么可以使用以下代码:
```
(def num-players 3)
(def hand1 [:As :Kd])
(def hand2 [:Qs :Jd])
(def hand-combinations (generate-all-hand-combinations cards num-players))
(def win-count (count (filter #(= 1 (calculate-win-probability hand1 hand2)) hand-combinations)))
(/ win-count (count hand-combinations))
```
在这个代码中,我们首先定义了参与游戏的人数和两个玩家的手牌。然后使用generate-all-hand-combinations函数生成所有可能的手牌组合。接下来,使用filter函数过滤出所有获胜的手牌组合,并统计它们的数量。最后,将获胜数量除以总数量,得到获胜概率。
阅读全文
相关推荐
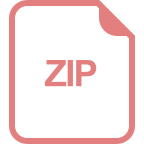
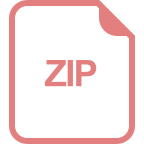
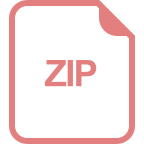







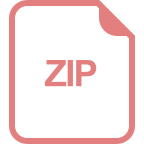
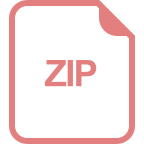
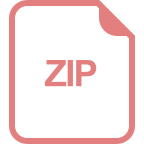
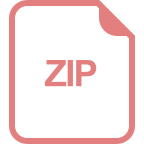
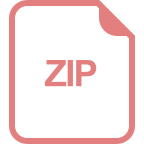
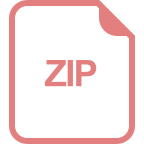
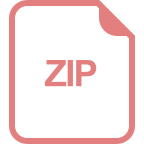
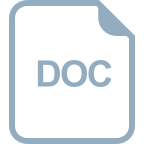
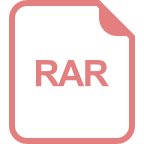
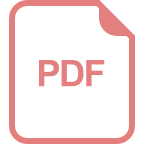