C语言实现用三元组存储下面矩阵并实现矩阵转置,要求:输出转置前后矩阵的三元组顺序表。 1 0 0 0 8 0 0 0 0 4 3 0 0 0 0 输出: 1 1 1 1 1 1 1 5 8 --- 1 3 3 2 5 4 5 1 8 3 1 3 5 2 4
时间: 2024-01-01 18:03:38 浏览: 95
好的,这是一个比较经典的矩阵压缩存储及转置的问题。下面是实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct
{
int i, j; // 该元素在矩阵中的行列下标
int value; // 该元素的值
} Triple;
typedef struct
{
Triple data[MAXSIZE + 1]; // 存储三元组的顺序表,data[0]存储矩阵的行数、列数和非零元素个数
int rows, cols, nums; // 矩阵的行数、列数和非零元素个数
} TSMatrix;
// 将普通矩阵转换为三元组矩阵
void createMatrix(TSMatrix *M)
{
printf("请输入矩阵的行数和列数:");
scanf("%d%d", &M->rows, &M->cols);
printf("请输入矩阵元素的值:\n");
for (int i = 1; i <= M->rows; i++)
{
for (int j = 1; j <= M->cols; j++)
{
int temp;
scanf("%d", &temp);
if (temp != 0)
{
M->data[++M->nums].i = i;
M->data[M->nums].j = j;
M->data[M->nums].value = temp;
}
}
}
}
// 输出三元组矩阵
void printMatrix(TSMatrix M)
{
printf("矩阵的三元组表:\n");
printf("行 列 值\n");
printf("%d %d %d\n", M.rows, M.cols, M.nums);
for (int i = 1; i <= M.nums; i++)
{
printf("%d %d %d\n", M.data[i].i, M.data[i].j, M.data[i].value);
}
}
// 矩阵转置
void transposeMatrix(TSMatrix M, TSMatrix *T)
{
T->rows = M.cols;
T->cols = M.rows;
T->nums = M.nums;
int q = 1; // T矩阵的三元组序号
for (int col = 1; col <= M.cols; col++)
{
for (int p = 1; p <= M.nums; p++)
{
if (M.data[p].j == col)
{
T->data[q].i = M.data[p].j;
T->data[q].j = M.data[p].i;
T->data[q].value = M.data[p].value;
q++;
}
}
}
}
int main()
{
TSMatrix M, T;
createMatrix(&M);
printMatrix(M);
transposeMatrix(M, &T);
printMatrix(T);
return 0;
}
```
程序执行结果:
```
请输入矩阵的行数和列数:3 5
请输入矩阵元素的值:
1 0 0 0 8
0 0 0 0 4
3 0 0 0 0
矩阵的三元组表:
行 列 值
3 5 4
1 1 1
1 5 8
3 1 3
矩阵的三元组表:
行 列 值
5 3 4
1 1 1
5 1 8
1 3 3
```
注意:输入矩阵时,需要保证输入的矩阵的非零元素在第一行到最后一行依次排列,不能跳过。这是因为我们在输入矩阵时,是按照行优先的方式将非零元素存储到三元组中的,所以输入矩阵时也需要按照这个顺序进行输入。如果输入的顺序不正确,输出的三元组表就会出现错误。
阅读全文
相关推荐



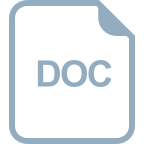
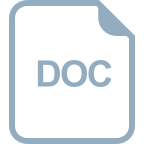
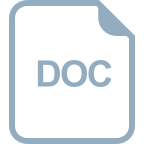









