大量多边形求交的C#实现
时间: 2023-07-12 19:01:36 浏览: 52
以下是使用 C# 实现大量多边形求交的示例代码:
```csharp
using System;
using System.Collections.Generic;
namespace PolygonIntersection
{
public class Point
{
public double X { get; set; }
public double Y { get; set; }
public Point(double x, double y)
{
X = x;
Y = y;
}
}
public class Edge
{
public Point Start { get; set; }
public Point End { get; set; }
public Edge(Point start, Point end)
{
Start = start;
End = end;
}
public bool Intersects(Edge other)
{
double x1 = Start.X, y1 = Start.Y, x2 = End.X, y2 = End.Y;
double x3 = other.Start.X, y3 = other.Start.Y, x4 = other.End.X, y4 = other.End.Y;
double denominator = (y4 - y3) * (x2 - x1) - (x4 - x3) * (y2 - y1);
if (denominator == 0)
return false;
double ua = ((x4 - x3) * (y1 - y3) - (y4 - y3) * (x1 - x3)) / denominator;
double ub = ((x2 - x1) * (y1 - y3) - (y2 - y1) * (x1 - x3)) / denominator;
return ua >= 0 && ua <= 1 && ub >= 0 && ub <= 1;
}
}
public class Polygon
{
public List<Point> Vertices { get; set; }
public Polygon(List<Point> vertices)
{
Vertices = vertices;
}
public bool Intersects(Polygon other)
{
List<Edge> edges1 = GetEdges();
List<Edge> edges2 = other.GetEdges();
foreach (Edge edge1 in edges1)
{
foreach (Edge edge2 in edges2)
{
if (edge1.Intersects(edge2))
return true;
}
}
return false;
}
private List<Edge> GetEdges()
{
List<Edge> edges = new List<Edge>();
for (int i = 0; i < Vertices.Count - 1; i++)
{
edges.Add(new Edge(Vertices[i], Vertices[i + 1]));
}
edges.Add(new Edge(Vertices[Vertices.Count - 1], Vertices[0]));
return edges;
}
}
class Program
{
static void Main(string[] args)
{
List<Point> vertices1 = new List<Point>()
{
new Point(0, 0),
new Point(0, 2),
new Point(2, 2),
new Point(2, 0)
};
List<Point> vertices2 = new List<Point>()
{
new Point(1, 1),
new Point(1, 3),
new Point(3, 3),
new Point(3, 1)
};
Polygon polygon1 = new Polygon(vertices1);
Polygon polygon2 = new Polygon(vertices2);
if (polygon1.Intersects(polygon2))
Console.WriteLine("Polygons intersect");
else
Console.WriteLine("Polygons do not intersect");
Console.ReadLine();
}
}
}
```
上述代码实现了 Point、Edge 和 Polygon 三个类,其中 Point 表示一个点,Edge 表示一条边,Polygon 表示一个多边形。Polygon 类中的 GetEdges 方法用于获取多边形的所有边,Intersects 方法用于判断多边形是否与另一个多边形相交。最后的示例代码中创建了两个多边形并判断其是否相交。
相关推荐
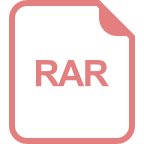














