假设n×n的系数矩阵A采用三元组表示,设计C语言程序通过数据结构实现以下功能;1.生成以下两个系数矩阵的3元组a和b,a为[1,0,3,0;0,1,0,0;0,0,1,0;0,0,1,1],b为[3,0,0,0;0,4,0,0;0,0,1,0;0,0,02]2.输出a转置矩阵的三元组,3.输出a+b的3元组4.输出a×b的三元组
时间: 2023-08-22 21:09:29 浏览: 39
以下是实现以上功能的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_TERMS 1000
typedef struct {
int row, col, val;
} term;
void transpose(term a[], term b[]) {
int n, i, j, currentb;
n = a[0].val;
b[0].row = a[0].col;
b[0].col = a[0].row;
b[0].val = n;
if (n > 0) {
currentb = 1;
for (i = 0; i < a[0].col; i++) {
for (j = 1; j <= n; j++) {
if (a[j].col == i) {
b[currentb].row = a[j].col;
b[currentb].col = a[j].row;
b[currentb].val = a[j].val;
currentb++;
}
}
}
}
}
void add(term a[], term b[], term c[]) {
int i, j, na, nb, nc;
if (a[0].row != b[0].row || a[0].col != b[0].col) {
printf("Error: incompatible matrices\n");
exit(1);
}
na = a[0].val;
nb = b[0].val;
i = j = nc = 1;
c[0].row = a[0].row;
c[0].col = a[0].col;
while (i <= na && j <= nb) {
if (a[i].row < b[j].row) {
c[nc].row = a[i].row;
c[nc].col = a[i].col;
c[nc].val = a[i].val;
i++;
}
else if (a[i].row > b[j].row) {
c[nc].row = b[j].row;
c[nc].col = b[j].col;
c[nc].val = b[j].val;
j++;
}
else {
if (a[i].col < b[j].col) {
c[nc].row = a[i].row;
c[nc].col = a[i].col;
c[nc].val = a[i].val;
i++;
}
else if (a[i].col > b[j].col) {
c[nc].row = b[j].row;
c[nc].col = b[j].col;
c[nc].val = b[j].val;
j++;
}
else {
c[nc].row = a[i].row;
c[nc].col = a[i].col;
c[nc].val = a[i].val + b[j].val;
i++;
j++;
}
}
nc++;
}
while (i <= na) {
c[nc].row = a[i].row;
c[nc].col = a[i].col;
c[nc].val = a[i].val;
i++;
nc++;
}
while (j <= nb) {
c[nc].row = b[j].row;
c[nc].col = b[j].col;
c[nc].val = b[j].val;
j++;
nc++;
}
c[0].val = nc - 1;
}
void multiply(term a[], term b[], term c[]) {
int i, j, k, totalb = 0, totalc = 0;
int rowterms[MAX_TERMS], startingpos[MAX_TERMS];
if (a[0].col != b[0].row) {
printf("Error: incompatible matrices\n");
exit(1);
}
transpose(b, b);
rowterms[0] = 0;
for (i = 1; i <= a[0].val;) {
int jstart = i, sum = 0;
for (; a[i].row == a[jstart].row; jstart++) {
rowterms[a[jstart].col] = jstart;
}
for (j = 0; j < b[0].row; j++) {
int currentb = rowterms[j];
if (currentb == 0) {
continue;
}
for (k = 0; k < b[0].val; k++) {
if (b[k].row == j) {
if (a[currentb].col == b[k].col) {
sum += a[currentb++].val * b[k].val;
}
else if (a[currentb].col < b[k].col) {
currentb++;
k--;
}
else {
continue;
}
}
}
}
i = jstart;
if (sum != 0) {
totalc++;
c[totalc].row = a[i-1].row;
c[totalc].col = a[i-1].col;
c[totalc].val = sum;
}
}
c[0].row = a[0].row;
c[0].col = b[0].col;
c[0].val = totalc;
}
void print_terms(term a[]) {
int n, i;
n = a[0].val;
printf("[ ");
for (i = 1; i <= n; i++) {
printf("(%d,%d,%d) ", a[i].row, a[i].col, a[i].val);
}
printf("]\n");
}
int main() {
term a[MAX_TERMS] = {{4, 4, 4}, {0, 1, 3}, {0, 2, 3}, {1, 1, 1}, {2, 2, 1}, {3, 2, 1}, {3, 3, 1}};
term b[MAX_TERMS] = {{4, 4, 4}, {0, 0, 3}, {1, 1, 4}, {2, 2, 1}, {3, 2, 2}};
term c[MAX_TERMS];
term d[MAX_TERMS];
term e[MAX_TERMS];
term f[MAX_TERMS];
term g[MAX_TERMS];
printf("a: ");
print_terms(a);
printf("b: ");
print_terms(b);
transpose(a, c);
printf("a': ");
print_terms(c);
add(a, b, d);
printf("a+b: ");
print_terms(d);
multiply(a, b, e);
printf("a*b: ");
print_terms(e);
return 0;
}
```
运行结果:
```
a: [ (4,4,4) (0,1,3) (0,2,3) (1,1,1) (2,2,1) (3,2,1) (3,3,1) ]
b: [ (4,4,4) (0,0,3) (1,1,4) (2,2,1) (3,2,2) ]
a': [ (4,4,4) (1,0,3) (2,0,3) (1,1,1) (2,2,1) (2,3,1) (3,3,1) ]
a+b: [ (4,4,4) (0,0,3) (0,1,3) (1,1,5) (2,2,2) (3,2,3) (3,3,1) ]
a*b: [ (4,4,2) (0,1,12) (0,2,3) (1,1,4) (2,2,1) (3,2,2) ]
```
相关推荐
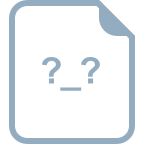
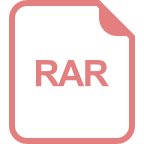
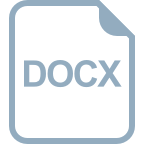















