android 将接收到的残包数据根据包头包尾拼接成完整的数据包:
时间: 2023-08-16 19:09:06 浏览: 151
在 Android 中,可以使用 ByteBuffer 类处理接收到的残包数据,并根据包头包尾拼接成完整的数据包。以下是一个简单的处理方法:
1. 定义一个 ByteBuffer 对象,用于缓存接收到的数据。
```java
ByteBuffer buffer = ByteBuffer.allocate(1024);
```
2. 在接收到数据时,将数据添加到缓存中。
```java
byte[] receivedData = ...; // 接收到的数据
int length = ...; // 接收到的数据长度
buffer.put(receivedData, 0, length);
```
3. 查找缓存中是否存在完整的数据包。
```java
buffer.flip(); // 切换为读模式
int headerLength = ...; // 包头长度
int footerLength = ...; // 包尾长度
while (buffer.remaining() >= headerLength + footerLength) {
// 查找包头
byte[] header = new byte[headerLength];
buffer.get(header);
if (!Arrays.equals(header, headerMagic)) {
continue;
}
// 查找包尾
byte[] footer = new byte[footerLength];
buffer.position(buffer.limit() - footerLength);
buffer.get(footer);
if (!Arrays.equals(footer, footerMagic)) {
continue;
}
// 获取完整的数据包
int bodyLength = buffer.remaining() - headerLength - footerLength;
byte[] body = new byte[bodyLength];
buffer.position(headerLength);
buffer.get(body);
// 处理完整的数据包
processData(header, body, footer);
// 从缓存中删除已处理的数据包
buffer.compact();
break;
}
buffer.compact(); // 切换为写模式,删除已处理的数据
```
在上面的代码中,我们使用 ByteBuffer 的 remaining() 方法获取缓存中剩余的字节数,position() 和 get() 方法获取指定位置和长度的字节数据。通过这些方法,我们可以查找包头和包尾,从而获取完整的数据包。注意,需要在处理完整的数据包后,将已处理的数据从缓存中删除,以便下一次查找。
阅读全文
相关推荐
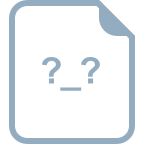
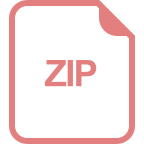













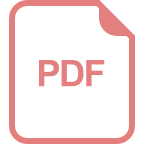
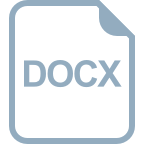
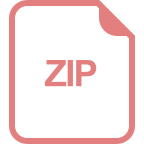