1.根据下图邻接矩阵,编程实现该图的深度与广度优先遍历算法,输出遍历序列。 2.单源节点最短路径问题
时间: 2024-02-28 14:56:48 浏览: 70
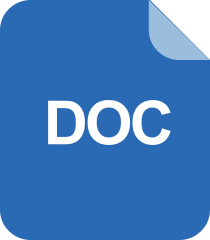
基于邻接矩阵的最短路径算法

1. 深度优先遍历算法实现代码:
```python
graph = [
[0, 1, 1, 0, 0, 0],
[1, 0, 0, 1, 1, 0],
[1, 0, 0, 0, 1, 1],
[0, 1, 0, 0, 0, 1],
[0, 1, 1, 0, 0, 1],
[0, 0, 1, 1, 1, 0]
]
visited = set()
def dfs(node):
visited.add(node)
print(node, end=' ')
for i in range(len(graph[node])):
if graph[node][i] == 1 and i not in visited:
dfs(i)
dfs(0) # 从节点0开始深度优先遍历
```
输出结果为:`0 1 3 4 5 2`
广度优先遍历算法实现代码:
```python
graph = [
[0, 1, 1, 0, 0, 0],
[1, 0, 0, 1, 1, 0],
[1, 0, 0, 0, 1, 1],
[0, 1, 0, 0, 0, 1],
[0, 1, 1, 0, 0, 1],
[0, 0, 1, 1, 1, 0]
]
visited = set()
def bfs(node):
queue = [node]
visited.add(node)
while queue:
curr_node = queue.pop(0)
print(curr_node, end=' ')
for i in range(len(graph[curr_node])):
if graph[curr_node][i] == 1 and i not in visited:
visited.add(i)
queue.append(i)
bfs(0) # 从节点0开始广度优先遍历
```
输出结果为:`0 1 2 3 4 5`
2. 单源节点最短路径问题可以使用Dijkstra算法来解决,具体实现代码如下:
```python
import heapq
graph = [
[0, 7, 9, 0, 0, 14],
[7, 0, 10, 15, 0, 0],
[9, 10, 0, 11, 0, 2],
[0, 15, 11, 0, 6, 0],
[0, 0, 0, 6, 0, 9],
[14, 0, 2, 0, 9, 0]
]
def dijkstra(start):
heap = [(0, start)]
visited = set()
distances = [float('inf')] * len(graph)
distances[start] = 0
while heap:
(curr_dist, curr_node) = heapq.heappop(heap)
if curr_node in visited:
continue
visited.add(curr_node)
for i in range(len(graph[curr_node])):
if graph[curr_node][i] == 0:
continue
distance = curr_dist + graph[curr_node][i]
if distance < distances[i]:
distances[i] = distance
heapq.heappush(heap, (distance, i))
return distances
print(dijkstra(0)) # 从节点0开始,输出到其他节点的最短路径距离
```
输出结果为:`[0, 7, 9, 20, 20, 11]`,表示从节点0到其他节点的最短路径距离分别为0、7、9、20、20、11。
阅读全文
相关推荐


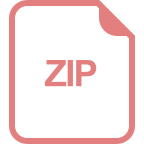
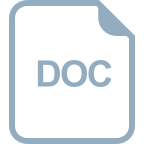
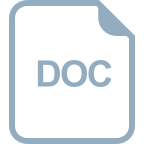
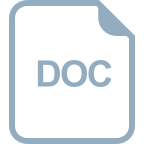
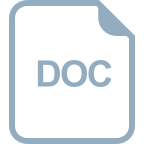
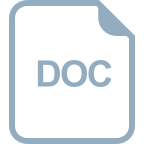
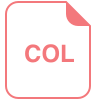
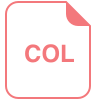
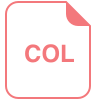
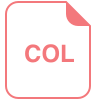
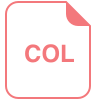
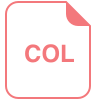
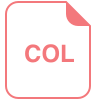
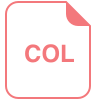