#include "iostream" #include "math.h" using namespace std; class coord{ private: double coord_x; double coord_y; public: coord() { coord_x = coord_y = 0; cout << "调用构造函数coord()" << endl; system("pause"); } ~coord() { cout << "调用析构函数~coord()" << endl; system("pause"); } void set_coord(double x,double y) { coord_x = x; coord_y = y; } double distance(coord &a,coord &b) { return sqrt((a.coord_x-b.coord_x)*(a.coord_x-b.coord_x)+(a.coord_y-b.coord_y)*(a.coord_y-b.coord_y)); } }; class circle{ private: double r; double c_x; double c_y; public: circle() { r=c_x=c_y=0; cout << "调用构造函数circle()" << endl; system("pause"); } ~circle() { cout << "调用析构函数~circle()" << endl; system("pause"); } void set_circle(double x,double y,double R) { if(r<=0) { cout << "error" << endl; exit(0); } r=R; c_x=x; c_y=y; } void if_intersect(circle& c1 , circle& c2) { double dist_1, dist_2; coord o1,o2; o1.set_coord(c1.c_x,c1.c_y); o2.set_coord(c2.c_x,c2.c_y); dist_1 = distance(o1,o2); dist_2 = c1.r + c2.r; if(dist_1<dist_2) cout << "相交" <<endl; else if(dist_1>dist_2) cout << "不相交" <<endl; else cout << "相切" <<endl; } }; int main() { circle c1,c2; double x1,y1,r1,x2,y2,r2; cout << "input the coord and radius of circle1(double) (x,y,r):"; cin >> x1 >> y1 >> r1; c1.set_circle(x1,y1,r1); cout << "input the coord and radius of circle2(double) (x,y,r):"; cin >> x2 >> y2 >> r2; c2.set_circle(x2,y2,r2); if_intersect(c1,c2); return 0; }
时间: 2023-06-16 11:07:34 浏览: 70
这是一个 C++ 的程序,实现了判断两个圆是否相交的功能。程序中定义了两个类,一个是 coord 类,表示坐标点;另一个是 circle 类,表示圆。在 circle 类中,使用了 coord 类来表示圆心坐标,并且实现了判断两个圆是否相交的功能。
程序中使用了构造函数和析构函数来初始化和清理对象。构造函数在创建对象时被调用,析构函数在对象被销毁时被调用。在本程序中,构造函数和析构函数被用来输出调试信息和等待用户按下任意键继续执行程序。
在 main 函数中,首先输入了两个圆的坐标和半径,然后创建了两个 circle 对象,分别表示这两个圆。最后调用 if_intersect 方法来判断这两个圆是否相交。
相关问题
解释代码#include <iostream> #include <cstdio> #include <cstdlib> #include <ctime> #include <conio.h> #include <cmath> #include <windows.h> using namespace std; HANDLE hout = GetStdHandle(STD_OUTPUT_HANDLE); COORD coord;
这段代码使用了一些 C++ 语言中的头文件和函数,具体解释如下:
- `#include <iostream>`:包含了标准输入输出流的库函数,如 `std::cout` 和 `std::cin`。
- `#include <cstdio>`:包含了 C 标准库的输入输出函数,如 `printf()` 和 `scanf()`。
- `#include <cstdlib>`:包含了 C 标准库的通用工具函数,如 `malloc()` 和 `free()`。
- `#include <ctime>`:包含了 C 标准库的时间和日期函数,如 `time()` 和 `asctime()`。
- `#include <conio.h>`:包含了控制台输入输出函数,如 `getch()` 和 `clrscr()`。
- `#include <cmath>`:包含了 C 标准库的数学函数,如 `sin()` 和 `cos()`。
- `#include <windows.h>`:包含了 Windows 系统编程相关的函数和数据类型,如 `HANDLE` 和 `COORD`。
其中,`HANDLE` 类型表示一个操作系统句柄,用于访问系统资源;`COORD` 类型表示控制台屏幕上的坐标。该代码段还定义了一个全局变量 `hout`,用于获取标准输出句柄,并定义了一个函数 `SetColor()`,用于设置控制台输出的文本颜色。
#include "iostream" #include "math.h" using namespace std; class coord{ private: double coord_x; double coord_y; public: coord() { coord_x = coord_y = 0; cout << "调用构造函数coord()" << endl; system("pause"); } ~coord() { cout << "调用析构函数~coord()" << endl; system("pause"); } void set_coord(double x,double y) { coord_x = x; coord_y = y; } double distance(coord &a,coord &b) { return sqrt((a.coord_x-b.coord_x)(a.coord_x-b.coord_x)+(a.coord_y-b.coord_y)(a.coord_y-b.coord_y)); } }; class circle{ private: double r; double c_x; double c_y; public: circle() { r=c_x=c_y=0; cout << "调用构造函数circle()" << endl; system("pause"); } ~circle() { cout << "调用析构函数~circle()" << endl; system("pause"); } void set_circle(double x,double y,double R) { if(r<=0) { cout << "error" << endl; exit(0); } r=R; c_x=x; c_y=y; } void if_intersect(circle& c1 , circle& c2) { double dist_1, dist_2; coord o1,o2; o1.set_coord(c1.c_x,c1.c_y); o2.set_coord(c2.c_x,c2.c_y); dist_1 = distance(o1,o2); dist_2 = c1.r + c2.r; if(dist_1<dist_2) cout << "相交" <<endl; else if(dist_1>dist_2) cout << "不相交" <<endl; else cout << "相切" <<endl; } }; int main() { circle c1,c2; double x1,y1,r1,x2,y2,r2; cout << "input the coord and radius of circle1(double) (x,y,r):"; cin >> x1 >> y1 >> r1; c1.set_circle(x1,y1,r1); cout << "input the coord and radius of circle2(double) (x,y,r):"; cin >> x2 >> y2 >> r2; c2.set_circle(x2,y2,r2); if_intersect(c1,c2); return 0; }
这是一个 C++ 程序,实现了判断两个圆是否相交或相切。程序中定义了两个类,分别是 coord 类和 circle 类。
coord 类表示坐标点,有两个私有成员变量 coord_x 和 coord_y,分别表示横坐标和纵坐标。类中包含一个构造函数和一个析构函数,还有一个公有成员函数 set_coord,用于设置坐标点的横纵坐标。此外,类中还有一个公有成员函数 distance,用于计算两个坐标点之间的距离。
circle 类表示圆,有三个私有成员变量 r、c_x 和 c_y,分别表示圆的半径、圆心的横坐标和纵坐标。类中包含一个构造函数和一个析构函数,还有一个公有成员函数 set_circle,用于设置圆的半径和圆心的横纵坐标。此外,类中还有一个公有成员函数 if_intersect,用于判断两个圆是否相交或相切。
在 main 函数中,先输入圆1和圆2的半径和圆心坐标,然后创建两个 circle 类的对象 c1 和 c2,并调用它们的 set_circle 函数设置半径和圆心坐标。最后调用 c1 的 if_intersect 函数,判断两个圆是否相交或相切。
阅读全文
相关推荐
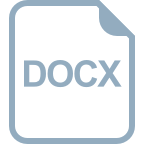
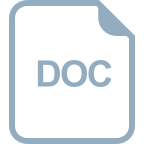
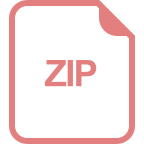












