用C++数组模拟栈完成:输入一行表示后缀表达式,注意每个数或符号之间有一空格隔开,最后输入 @ 表示输入结束。(给定的表达式不超过 1000 个字符) 注意:本题中遇到除法运算,只需要计算整除的结果,不需要保留小数。
时间: 2024-05-19 20:17:35 浏览: 76
好的,我理解了你的要求。以下是代码:
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_SIZE 1000
int stack[MAX_SIZE];
int top = -1;
void push(int value)
{
if (top == MAX_SIZE - 1)
{
printf("Stack is full!\n");
exit(1);
}
stack[++top] = value;
}
int pop()
{
if (top == -1)
{
printf("Stack is empty!\n");
exit(1);
}
return stack[top--];
}
int main()
{
char expression[MAX_SIZE];
printf("Please enter the postfix expression (separated by spaces):\n");
fgets(expression, MAX_SIZE, stdin);
int i = 0, num = 0;
char c;
while ((c = expression[i++]) != '@')
{
if (isspace(c)) // ignore spaces
continue;
if (isdigit(c))
{
num = num * 10 + (c - '0');
}
else if (c == '+')
{
int b = pop();
int a = pop();
push(a + b);
}
else if (c == '-')
{
int b = pop();
int a = pop();
push(a - b);
}
else if (c == '*')
{
int b = pop();
int a = pop();
push(a * b);
}
else if (c == '/')
{
int b = pop();
int a = pop();
push(a / b);
}
else
{
push(num);
num = 0;
}
}
printf("Result: %d", pop());
return 0;
}
阅读全文
相关推荐
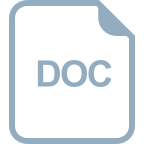
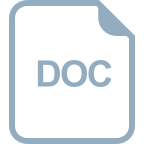
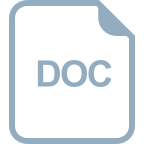





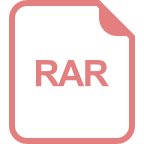









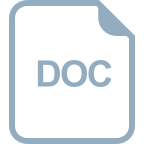